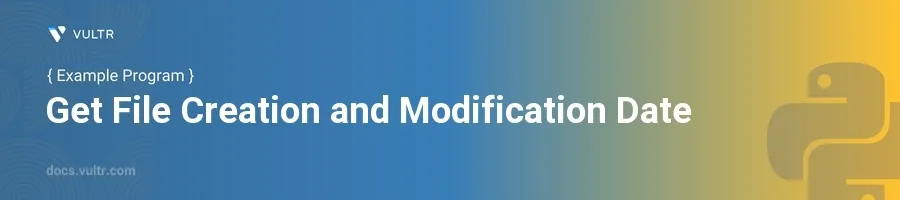
Introduction
Working with file metadata in Python, such as obtaining file creation and modification dates, is a common need for many automation, logging, and data management tasks. Whether you're organizing files, checking for updates, or just collecting information, Python offers straightforward tools to access this data.
In this article, you will learn how to retrieve the creation and modification dates of files using Python. Explore how to handle these tasks across different operating systems, such as Windows, macOS, and Linux, emphasizing cross-platform solutions and common pitfalls.
Retrieving File Dates in Python
When working with files in Python, you can use the os
module to perform system-related file operations. However, for date-related information, you will typically make use of the os.path
and time
modules.
Get Modification Date
The modification date of a file is the last time the file was altered. To retrieve this information:
Import the required modules.
Specify the path to the file.
Use the
os.path.getmtime()
method.pythonimport os import time file_path = 'example.txt' modification_time = os.path.getmtime(file_path) readable_time = time.ctime(modification_time) print(f'Last modification time: {readable_time}')
This script converts the timestamp returned by
getmtime()
into a human-readable format usingctime()
from thetime
module.
Get Creation Date
Retrieving the creation date is straightforward on Windows but can be less intuitive on Unix-like systems (macOS and Linux), where typically the "change" time is available.
For Windows, use
os.path.getctime()
for the creation time.For Unix-like systems, the creation time concept does not directly apply;
os.path.getctime()
returns the time of the last metadata change.pythoncreation_time = os.path.getctime(file_path) readable_creation_time = time.ctime(creation_time) print(f'Creation time: {readable_creation_time}')
This provides the creation time on Windows and the last metadata change time on Unix-like systems.
Handling Time on Different Operating Systems
File time metadata behaves differently across operating systems. This section clarifies these differences and suggests how to handle them effectively.
Windows
Windows tracks file creation, last access, and last modification times directly:
- Use
getctime()
for creation time as shown previously. - Alternatively, use additional libraries like
pywin32
to access more specific file attributes if needed.
Unix (macOS and Linux)
These systems do not typically track the actual creation time:
- The returned "creation time" from
os.path.getctime()
is actually the time of last inode change. - If true creation time is needed, consider using the filesystem-specific commands or debugging tools, which might not be available through Python directly.
Cross-platform Considerations
To write a cross-platform script that handles file times, perform checks within your Python script to determine the operating system, and adjust your logic accordingly:
- Use the
platform
module to determine the running OS. - Based on the OS, decide which attributes to fetch, as shown in the examples above.
Conclusion
Retrieving file creation and modification dates in Python is essential for several applications, from file management to data analytics. While the process is straightforward in Windows, Unix-like operating systems require special consideration due to their different handling of file system metadata. By utilizing the os.path
and time
modules, you can write effective, cross-platform scripts that access and use file date metadata to fulfill your programming needs. Equip your programming toolkit with these methods to manage and utilize file metadata efficiently in any Python application.
No comments yet.