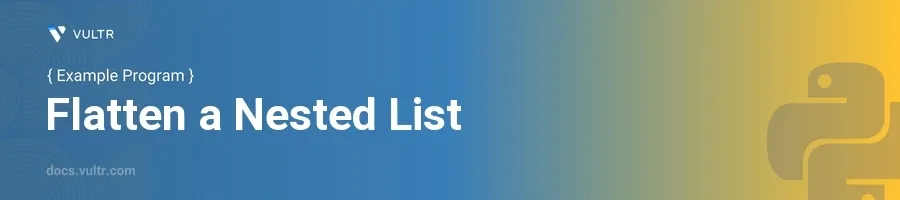
Introduction
Dealing with nested lists in Python is a common task, especially in applications involving data processing or manipulation. Nested lists, or lists within lists, can present challenges when you need to perform operations that require a flat list. Flattening a nested list means converting it into a single list that contains all the elements of the nested lists at one level, without any sub-lists.
In this article, you will learn how to flatten a nested list using different methods in Python. You’ll explore multiple examples that demonstrate practical approaches, including iterative methods and recursion. By the end, you will be well-equipped to handle any nested list structure you might encounter in your coding tasks.
Flattening Using a Loop
Simple Loop with List Comprehension
For moderately nested lists or when the depth of the list is known and consistent, a simple loop with a list comprehension can be quite effective.
Begin with a nested list.
Use a list comprehension to iterate through each element.
Check if the element is a list. If so, extend the flat list by the element; otherwise, append the element directly.
pythonnested_list = [[1, 2, [3, 4]], [5, 6], 7] def flatten_list(nlist): flat_list = [] for item in nlist: if isinstance(item, list): flat_list.extend(flatten_list(item)) else: flat_list.append(item) return flat_list print(flatten_list(nested_list))
This solution applies a recursive approach to handle lists with varying depths. It checks whether each item is a list and calls itself recursively when required, seamlessly managing multiple levels of nesting.
Using Recursion
Deep Flattening with Recursive Function
When dealing with highly nested or irregularly nested lists, recursive functions shine by handling complexity without repetitive code structure.
Create a function that accepts a nested list.
Initialize an empty list to hold flattened results.
Traverse each item in the list: apply the function recursively if the item is a list; if not, append it to the result list.
pythondef recursive_flatten(nlist): result = [] for item in nlist: if isinstance(item, list): result.extend(recursive_flatten(item)) else: result.append(item) return result nested_list = [[1, 2, [3, [4, 5]]], 6] print(recursive_flatten(nested_list))
This code demonstrates a deep nesting level where the list contains other lists at different depths. It systematically flattens these structures into a simple, one-dimensional list.
Utilizing Python Libraries
Using itertools.chain
Python’s itertools
module provides a method called chain
, which can be helpful, especially in combination with itertools.chain.from_iterable
to flatten lists that are only one level deep.
Import
chain.from_iterable
fromitertools
.Place the nested list into
chain.from_iterable
to flatten it.pythonfrom itertools import chain nested_list = [[1, 2], [3, 4], [5, 6]] flat_list = list(chain.from_iterable(nested_list)) print(flat_list)
The
chain.from_iterable
function efficiently handles lists where each sublist is one level deep. It's not suited for deeply nested lists but works excellently for single-level nested lists.
Using Generators for Large Nested Lists
Generator Approach for Memory Efficiency
Generators offer a way to handle large nested lists without loading the entire list into memory.
Define a generator function that yields elements from a nested list one by one.
Use a
for
loop within the function to handle list items recursively.pythondef flatten_generator(nlist): for item in nlist: if isinstance(item, list): yield from flatten_generator(item) else: yield item nested_list = [[1, 2, [3, 4]], [5], [6, [7, 8, 9]]] flat_list = list(flatten_generator(nested_list)) print(flat_list)
With generators, you manage data flow efficiently, especially suitable for processing large datasets that might not fit into memory as a whole.
Conclusion
Flattening nested lists in Python is a task you can approach from multiple angles depending on the specific requirements of your application—whether it's handling basic shallow structures or complex and large data sets. From iterative methods to recursive functions and powerful Python library features, choose the method that best fits your situation. Armed with these strategies, make sure your applications handle nested lists with efficiency and ease.
No comments yet.