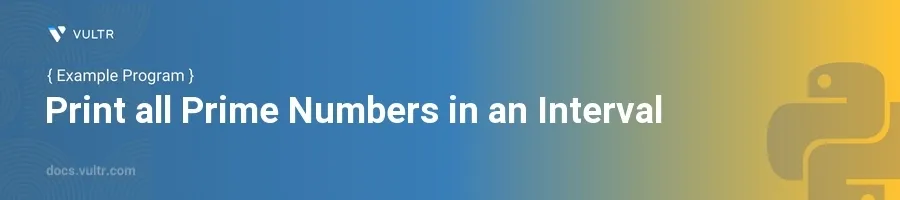
Introduction
Prime numbers, the building blocks of arithmetic, are intriguing not only to mathematicians but also to programmers. A prime number is a natural number greater than 1 that cannot be formed by multiplying two smaller natural numbers. In programming, generating prime numbers efficiently is a common challenge that tests a programmer's ability to implement algorithms.
In this article, you will learn how to create a Python program that prints all prime numbers in a given interval. Discover different methods to achieve this, including a straightforward approach and a more efficient technique using the Sieve of Eratosthenes.
Simple Approach to Find Prime Numbers
Define the Function
Create a function
is_prime(n)
that checks whether a number is prime.Use this function to print prime numbers in a specified interval.
pythondef is_prime(n): if n <= 1: return False for i in range(2, int(n**0.5) + 1): if n % i == 0: return False return True def print_primes(start, end): for number in range(start, end + 1): if is_prime(number): print(number, end=' ')
is_prime(n)
: Checks each number from 2 up to the square root ofn
. Ifn
is divisible by any number in this range, it's not a prime.print_primes(start, end)
: Loops through each number in the interval fromstart
toend
, inclusive, and prints the number if it's prime.
Execute the Function
Call
print_primes
with the interval you want to check.pythonprint_primes(10, 50)
This script prints all prime numbers between 10 and 50. The simple method checks each number individually, which is comprehensible but not the most efficient for large numbers or wide ranges.
Efficient Approach Using the Sieve of Eratosthenes
Implementing the Sieve Algorithm
Understand that the Sieve of Eratosthenes allows finding all prime numbers up to
n
efficiently.Implement the Sieve method and use it to find primes in an interval.
pythondef sieve_of_eratosthenes(n): is_prime = [True] * (n+1) p = 2 while (p * p <= n): if (is_prime[p] == True): for i in range(p * p, n+1, p): is_prime[i] = False p += 1 return is_prime def print_primes_with_sieve(start, end): is_prime = sieve_of_eratosthenes(end) for number in range(start, end + 1): if is_prime[number]: print(number, end=' ')
sieve_of_eratosthenes(n)
: Marks non-prime numbers asFalse
in a list indexed by numbers up ton
.- When called with
end
as the upper limit,is_prime
stores the primality of each number up toend
. print_primes_with_sieve(start, end)
: Uses the boolean list returned bysieve_of_eratosthenes
to print all primes in the specified interval.
Run the Efficient Sieve Function
Execute the function with your specified interval to see faster prime generation.
pythonprint_primes_with_sieve(10, 50)
This function call prints all primes between 10 and 50 much faster than the simple method, particularly beneficial when dealing with larger numbers or when needing to identify primes in extensive numerical ranges frequently.
Conclusion
Exploring different methods to print all prime numbers within an interval demonstrates the trade-offs between simplicity and efficiency in programming. While the straightforward method is easier to understand and implement, the Sieve of Eratosthenes provides a more efficient solution for larger scales, reducing the computational overhead significantly. Equipped with these techniques, you can choose the appropriate method based on the problem's scale and complexity to maintain both performance and clarity in your Python programs.
No comments yet.