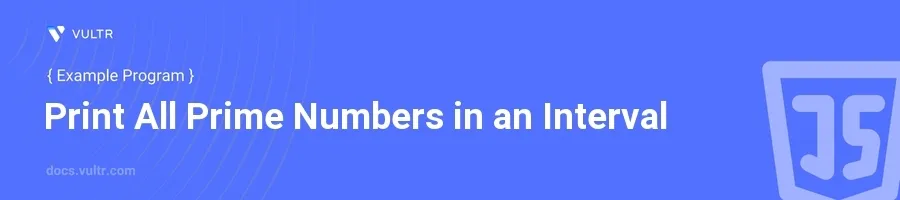
Introduction
Prime numbers are integers greater than 1 that have no divisors other than 1 and themselves. Finding all prime numbers within a specific interval is a common problem in programming, particularly for those learning algorithms and JavaScript. This task not only helps in understanding basic looping and conditional constructs but also aids in learning how to efficiently solve problems.
In this article, you will learn how to create a JavaScript program to find all prime numbers within a given interval. You'll explore examples of how to implement this, understand the logic behind prime checks, and see how loops and condition handling work in JavaScript.
JavaScript Program to Identify Prime Numbers
Define Prime Number Checking Function
Create a function to determine if a number is prime.
Inside the function, handle edge cases for numbers less than 2.
Implement a loop to check if the number can be divided by any number other than 1 and itself.
javascriptfunction isPrime(num) { if (num <= 1) return false; for (let i = 2; i * i <= num; i++) { if (num % i === 0) return false; } return true; }
This function starts by dismissing all numbers less than or equal to 1 as they are not prime. The loop checks for factors only up to the square root of the number (
i * i <= num
) because a larger factor ofnum
must be a multiple of a smaller factor that has already been checked.
Print Primes in a Given Interval
Define a function to print all prime numbers within an interval.
Use a loop to traverse through each number in the given interval.
Call the previously defined
isPrime
function to check for primality.Print numbers that are identified as prime.
javascriptfunction printPrimes(start, end) { for (let num = start; num <= end; num++) { if (isPrime(num)) { console.log(num); } } }
In this function, a loop runs from
start
toend
, inclusive, checking each number to determine if it's prime using theisPrime
function. If a number is prime, it’s printed to the console.
Example: Print Primes Between 10 and 50
Invoke the
printPrimes
function specifying the range as 10 to 50.javascriptprintPrimes(10, 50);
When called, this code will output all the prime numbers between 10 and 50 in the console.
Conclusion
The ability to find all prime numbers within a specified interval is a key skill in JavaScript programming that enhances your understanding of loops, conditionals, and function use. By implementing the isPrime
and printPrimes
functions demonstrated, you effectively handle the task of prime number detection within any range. Employ these strategies in your projects to improve your problem-solving skills and JavaScript proficiency.
No comments yet.