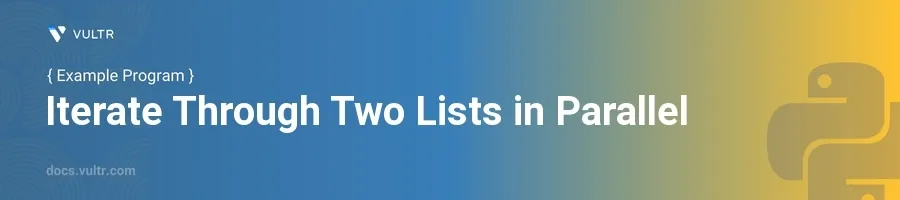
Introduction
Iterating through two lists in parallel is a common requirement in various programming scenarios where data correlation and manipulation of multiple data sets simultaneously are needed. This is especially useful in data analysis, synchronization tasks, and when performing operations that require elements from corresponding indexes in two or more lists.
In this article, you will learn how to iterate through two lists in parallel using Python. Explore several methods including the use of the built-in zip()
function, the zip_longest()
function from the itertools module for lists of unequal length, and a comparative discussion on performance considerations.
Using the zip() Function
Basic Parallel Iteration
Define two lists of equal length.
Use the
zip()
function to iterate through both lists simultaneously.pythonfruits = ["apple", "banana", "cherry"] colors = ["red", "yellow", "dark red"] for fruit, color in zip(fruits, colors): print(f"Fruit: {fruit}, Color: {color}")
This code pairs each element from the two lists (
fruits
andcolors
) and iterates through these pairs. Thezip()
function stops at the shortest list if they are not of equal length.
Handling Different Data Types
Recognize that
zip()
can handle any iterable types.Combine lists containing different data types.
pythonnames = ["Alice", "Bob", "Cathy"] scores = [85, 90, 88] for name, score in zip(names, scores): print(f"{name} scored {score}")
In this example, strings from
names
are paired with integers fromscores
, demonstratingzip()
's flexibility with different iterable data types.
Using itertools.zip_longest()
Iteration Over Lists of Unequal Length
Import the
zip_longest()
function from theitertools
module.Use
zip_longest()
when lists are of different lengths, providing a default value for missing elements.pythonfrom itertools import zip_longest fruits = ["apple", "banana", "cherry", "date"] colors = ["red", "yellow", "dark red"] for fruit, color in zip_longest(fruits, colors, fillvalue="No Color"): print(f"Fruit: {fruit}, Color: {color}")
zip_longest()
fills in with "No Color" for elements beyond the length of the shorter list (colors
in this case), allowing for full iteration of the longer list.
Performance Considerations
Efficiency of Iteration Techniques
- Understand that
zip()
is generally faster for lists of equal length due to its straightforward nature and direct stop mechanism when the shortest list ends. - Recognize that
zip_longest()
incurs slight additional overhead due to handling lists of differing lengths and filling in missing values.
It is important to choose the method that best suits the need of the task—opt for zip()
when working with lists of known equal length to maximize efficiency, or zip_longest()
when dealing with lists of varying lengths and a need to process all elements.
Conclusion
Iterating through two or more lists in parallel facilitates efficient and elegant handling of related data sets in Python. Whether the lists are of equal length or not, Python provides robust built-in tools, namely zip()
and zip_longest()
, to perform such tasks. Fully leveraging these functions not only simplifies the code but also enriches program logic by maintaining readability and performance. Adapt these methods to fit specific use cases and improve data handling capabilities in Python projects.
No comments yet.