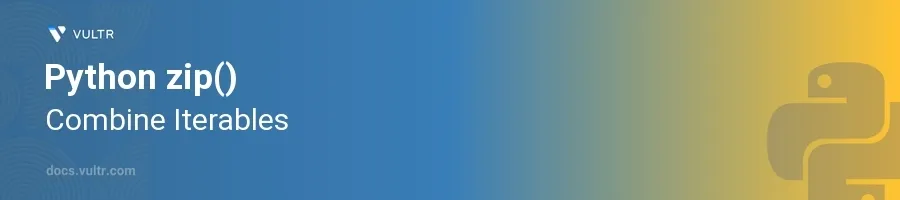
Introduction
The zip()
function in Python is a built-in utility that aggregates elements from each of the iterables provided. This function makes it easier to loop over multiple lists or iterables simultaneously, allowing for concise and readable code, especially when dealing with related data sets. Common uses include creating dictionaries from two separate lists of keys and values, or for iterating over multiple sequences at once in a single loop.
In this article, you will learn how to effectively harness the zip()
function in various scenarios. Discover how this function can simplify tasks when working with multiple data collections and explore its integration in data manipulation, looping constructs, and condition checks.
Combining Lists with zip()
Pair Elements from Two Lists
Start by defining two lists of equal length.
Apply the
zip()
function to pair elements from these lists.pythonlist1 = ['apple', 'banana', 'cherry'] list2 = [1, 2, 3] paired_list = list(zip(list1, list2)) print(paired_list)
This code combines corresponding elements from
list1
andlist2
into tuple pairs, resulting in a new listpaired_list
.
Multiple Iterables
Remember that
zip()
can handle more than two iterables.Define multiple lists and use
zip()
to combine them.pythonnames = ['Alice', 'Bob', 'Cindy'] ages = [25, 30, 35] cities = ['New York', 'Los Angeles', 'Chicago'] combined = list(zip(names, ages, cities)) print(combined)
In this example, each element from the lists
names
,ages
, andcities
is grouped together in tuples, providing a structured way to handle related multi-dimensional data.
Using zip() with Dictionaries
Creating a Dictionary from Two Lists
Define two lists, one for keys and one for values.
Use
zip()
to pair elements, then convert to a dictionary.pythonkeys = ['name', 'age', 'city'] values = ['Alice', 25, 'New York'] dict_from_lists = dict(zip(keys, values)) print(dict_from_lists)
This approach transforms paired elements from the lists
keys
andvalues
into key-value pairs within a dictionary,dict_from_lists
.
Updating Multiple Dictionaries
Combine multiple dictionaries by key using
zip()
.Iterate over paired key-value tuples to update or process.
pythondict1 = {'x': 1, 'y': 2} dict2 = {'x': 3, 'y': 4} for (k1, v1), (k2, v2) in zip(dict1.items(), dict2.items()): print(f"Sum of values for {k1}: {v1 + v2}")
Each iteration accesses pairs of items from
dict1
anddict2
, enabling operations on corresponding elements such as summing values with the same keys.
Advanced Uses of zip()
Transpose a Matrix
Understand that
zip()
can be instrumental in matrix computation.Use
zip(*matrix)
to transpose rows and columns.pythonmatrix = [ [1, 2, 3], [4, 5, 6], [7, 8, 9] ] transposed = list(zip(*matrix)) print("Transposed Matrix:", transposed)
The asterisk
*
operator unpacks the listmatrix
, andzip()
then recombines the elements as columns, effectively transposing the matrix.
Conclusion
The zip()
function in Python provides a highly convenient way to combine elements from multiple iterables, enhancing the efficiency and readability of your code. Whether pairing elements into tuples, forming dictionaries, or handling complex data structures, zip()
can significantly streamline your data operations. By mastering the techniques explored in this article, you ensure your code is not only functional but also clean and efficient when managing multiple data sets.
No comments yet.