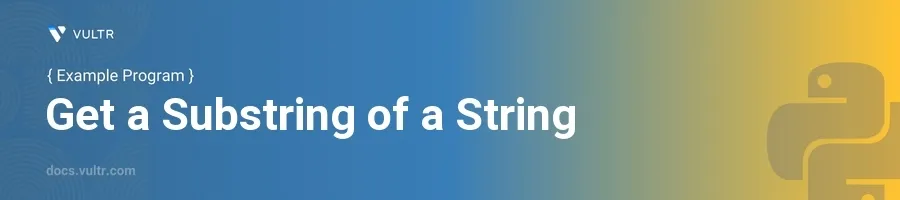
Introduction
In Python, extracting a substring from a string is a common operation that's straightforward to perform. This capability is ubiquitous in data parsing, manipulation, and conditional checks in various applications, from web development to data analysis. Understanding how to efficiently extract substrings is essential for any developer or analyst working with Python.
In this article, you will learn how to retrieve substrings from a string using different methods in Python. Detailed examples will demonstrate the use of slicing, the substr()
method, and regular expressions to achieve this goal with clarity and efficiency.
Extracting Substrings Using Slicing
Basic Slicing
Learn that slicing is the easiest way to extract a substring.
Specify the start index and the end index to slice the string.
pythonfull_text = "Hello, world!" substring = full_text[7:12] print(substring)
This code will print
world
. Slicing starts from the 7th character up to, but not including, the 12th character.
Slicing with Negative Indices
Understand that negative indices can be used to slice strings from the end.
Use a negative start index to get a substring starting from the end.
pythonfull_text = "Hello, world!" substring = full_text[-6:-1] print(substring)
Here, the substring
world
is extracted using negative indices.-6
starts the slice six characters from the end, up to, but not including, the last character.
Using the substring() Method
Extracting with substring() Function
Note: Python does not have a built-in substring()
method similar to other programming languages. However, you can achieve the same functionality using slicing or you can define a custom substring()
function.
Define a custom
substring()
function to emulate other languages.Use this function to specify start and end positions.
pythondef substring(value, start, end=None): if end is None: return value[start:] else: return value[start:end] full_text = "Hello, world!" print(substring(full_text, 0, 5)) print(substring(full_text, 7))
This will output:
Hello world!
Utilizing Regular Expressions for Substrings
Simple Match Extraction
Import the
re
module, which supports regular expressions.Use regular expressions to specify a pattern for the substring.
pythonimport re full_text = "Hello, world!" match = re.search(r"\bworld\b", full_text) if match: print(match.group(0))
This code prints
world
. It searches for the word "world" as a whole word within the string.
Complex Pattern Matching
Handle more complex patterns using regular expressions.
Extract parts of strings that match specific criteria.
pythonimport re full_text = "Contact us at: info@example.com" match = re.search(r"[\w\.-]+@[\w\.-]+", full_text) if match: print(match.group(0))
This code identifies email addresses in the string and extracts
info@example.com
. Regular expressions are potent for pattern matching and substring extraction in data validation tasks.
Conclusion
Extracting substrings is a routine task in Python programming, crucial in various domains such as text preprocessing, data validation, and pattern recognition. In this guide, you've learned to utilize Python's string slicing technique, a custom substring()
function, and regular expressions to precisely and efficiently extract substrings. Implement these methods according to your needs to manipulate and analyze strings effectively in your Python programs.
No comments yet.