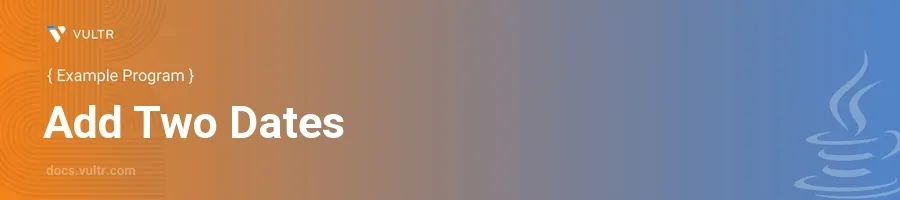
Introduction
Adding two dates in Java is a common requirement in many programming and application development scenarios, especially in areas like finance, scheduling, and logistics applications where date manipulation is frequently required. This task can be achieved through a variety of Java libraries and classes designed to handle date and time operations effectively.
In this article, you will learn how to add two dates in Java using different examples. Explore various methods to perform this operation effectively, applying both legacy classes like java.util.Date
and newer classes from the Java 8 Date-Time API like java.time.LocalDate
.
Example: Using java.util.Date and java.util.Calendar
Add Days to a Date
Import the necessary classes.
Create an instance of
java.util.Date
to represent the starting date.Utilize
java.util.Calendar
to add a specified number of days to the date.javaimport java.util.Calendar; import java.util.Date; public class DateAdder { public static void main(String[] args) { Date currentDate = new Date(); // Current date int daysToAdd = 10; // Days to add Calendar calendar = Calendar.getInstance(); calendar.setTime(currentDate); calendar.add(Calendar.DAY_OF_MONTH, daysToAdd); Date newDate = calendar.getTime(); System.out.println("New Date: " + newDate); } }
Here,
Calendar.getInstance()
obtains a calendar using the default time zone and locale. The calendar is then set tocurrentDate
, and days are added usingCalendar.add()
. ThenewDate
will have the additional days included.
Adding Months and Years
Follow similar steps to add different time units like months and years using
Calendar
.javacalendar.add(Calendar.MONTH, 5); calendar.add(Calendar.YEAR, 2);
These lines add 5 months and 2 years to the
currentDate
, respectively.
Example: Using Java 8 Date-Time API
Using LocalDate to Add Days, Months, or Years
Import
java.time.LocalDate
.Initialize a
LocalDate
object.Use
plusDays
,plusMonths
, orplusYears
methods to add time units.javaimport java.time.LocalDate; public class DateAdderJava8 { public static void main(String[] args) { LocalDate currentDate = LocalDate.now(); // Current date LocalDate newDate = currentDate.plusDays(10).plusMonths(2).plusYears(1); System.out.println("New Date: " + newDate); } }
This approach is cleaner and more intuitive than using the older
Calendar
class.LocalDate
represents a date without time-of-day or time-zone, which can vastly simplify day-to-day date handling.
Conclusion
Adding two dates in Java can be handled efficiently using either classic approaches with java.util.Calendar
or more modern methods provided by the Java 8 Date-Time API. The choice of method will largely depend on your specific requirements and the minimum Java version you support. Learn to harness these techniques to handle date manipulations adeptly within your Java applications, ensuring that you provide accurate calculations and robust functionalities.
No comments yet.