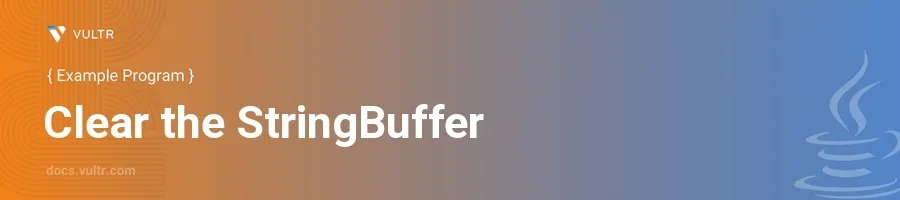
Introduction
In Java, the StringBuffer
class is used to create mutable strings, which means the contents of the strings can be modified after they are created. This provides a significant advantage in scenarios where a lot of string manipulation is required, reducing the need to create new string objects in memory for every modification.
In this article, you will learn how to clear the contents of a StringBuffer
instance in Java through practical examples. You'll explore methods such as setting its length to zero and using the delete
method to effectively reset a StringBuffer
.
Clearing StringBuffer Using setLength()
Set Length to Zero
Initialize a
StringBuffer
with some text.Use the
setLength(0)
method to clear its content.javaStringBuffer buffer = new StringBuffer("Hello, World!"); buffer.setLength(0); System.out.println("Buffer after clear: '" + buffer + "'");
This code initializes a
StringBuffer
with "Hello, World!" and then sets its length to 0, effectively clearing the buffer. When printed, the buffer shows as empty.
Verify the Buffer’s Capacity
Check the capacity before and after clearing the
StringBuffer
.javaStringBuffer buffer = new StringBuffer("Hello, World!"); System.out.println("Capacity before clear: " + buffer.capacity()); buffer.setLength(0); System.out.println("Capacity after clear: " + buffer.capacity());
In this example, the capacity of the
StringBuffer
before and after clearing is displayed. Notably, setting the length to zero does not change the capacity; it only affects the length.
Clearing StringBuffer Using delete()
Use delete() Method
Create a
StringBuffer
containing some text.Apply the
delete()
method to remove all content.javaStringBuffer buffer = new StringBuffer("Sample Text"); buffer.delete(0, buffer.length()); System.out.println("Buffer after delete: '" + buffer + "'");
The
delete()
method takes two parameters: the start and the end index. Here, it removes all characters from index 0 to the length of the buffer, clearing its contents.
Resetting Repeatedly in a Loop
Employ
delete()
in a loop for repetitive clearance tasks in scenarios such as text processing.javaStringBuffer buffer = new StringBuffer("Iteration "); int limit = 5; for (int i = 1; i <= limit; i++) { buffer.append(i); System.out.println(buffer); buffer.delete(0, buffer.length()); }
This snippet demonstrates using
StringBuffer
in a scenario where it needs to be cleared repeatedly within a loop, here appending and then erasing numerical increments.
Conclusion
Clearing a StringBuffer
in Java is straightforward and can be efficiently managed by using setLength(0)
or delete()
. Each approach has its use depending on the context and the specific requirement of the program. The setLength()
method is excellent for quick resets, while delete()
offers precise control over the range of text to be removed. By grasping these techniques, you enhance your ability to manage dynamic string operations effectively in Java applications.
No comments yet.