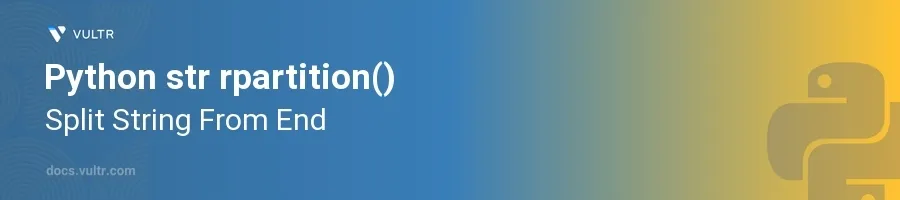
Introduction
The str.rpartition()
method in Python is a string manipulation tool that splits a string into a tuple, working from the end of the string towards the start. This function is especially useful for cases where you need to locate and partition around the last occurrence of a specific separator in a string. Unlike the str.partition()
method, which operates from the start, rpartition()
ensures that the partitioning focuses on the last (rightmost) segment, which can be particularly useful in file path manipulations, URL parsing, and more.
In this article, you will learn how to effectively utilize the str.rpartition()
method in various programming scenarios. Explore how this method can be used to separate filenames from their paths, handle reversed string operations, and efficiently manage data strings that require partitioning from the end.
Understanding the str.rpartition() Method
Basic Usage of str.rpartition()
Recognize that
str.rpartition()
takes a single argument: the separator string.Use
str.rpartition()
to divide a string starting from the rightmost occurrence of the separator.pythontext = "hello-world-example" partitioned = text.rpartition('-') print(partitioned)
In this code,
text.rpartition('-')
splits the string at the last occurrence of'-'
. The output will be a tuple:('hello-world', '-', 'example')
.
When the Separator is Not Found
Understand that if the specified separator is not found,
str.rpartition()
will still return a tuple.The tuple will contain two empty strings, and the original string as the third element.
pythontext = "hello.world.example" partitioned = text.rpartition('-') print(partitioned)
Here, since
'-'
is not found, the output will be:('', '', 'hello.world.example')
.
Handling File Paths and URLs
Using str.rpartition() to Handle File Paths
Consider extracting the filename from a full file path using the last occurrence of a file separator (e.g., '/').
Apply
str.rpartition()
for such scenarios.pythonfile_path = "/home/user/docs/report.txt" head, sep, tail = file_path.rpartition('/') print("Folder Path:", head) print("File Name:", tail)
This snippet partitions the
file_path
at the last slash, effectively separating the path and the file name.head
contains the directory path, andtail
gives the file name.
Using str.rpartition() for URLs
Utilize
str.rpartition()
to extract parameters or last segments from a URL.This method can help in cleanly stripping query parameters or file extensions.
pythonurl = "http://example.com/image.jpg?size=large" base, sep, query = url.rpartition('?') print("Base URL:", base) print("Query String:", query)
This simple operation splits the URL into the base part and the query string based on the last occurrence of
'?'
.
Practical Applications and Efficiency
Reversing String Operations
Use
str.rpartition()
methodically in reversed counterparts of common string operations.This is particularly useful in backward parsing, where reverse operations are more straightforward with
rpartition()
.pythonsentence = "This is a sentence. And another sentence." last_part = sentence.rpartition('.')[0] print(last_part)
Here, partitioning from the end helps to effectively isolate sections of a sentence or phrases before the last period.
Performance Considerations
- Note that
str.rpartition()
can sometimes offer performance benefits over similar operations because of its straight-to-the-point action on strings. - It avoids extra steps when only the last occurrence of a separator is significant for the string operation being performed.
Conclusion
The str.rpartition()
function in Python offers a robust way of handling string splits from the end towards the beginning. It's an invaluable tool where the focus is on parsing and managing strings constrained by the last occurrence of a specified character or substring. By addressing specific scenarios such as file path manipulations and URL parsing, str.rpartition()
not only simplifies code but also boosts its efficiency. Utilize this method in your scripts to maintain both readable and effective code. Through the examples and strategies discussed, this method should now integrate seamlessly into various aspects of your data handling and processing needs.
No comments yet.