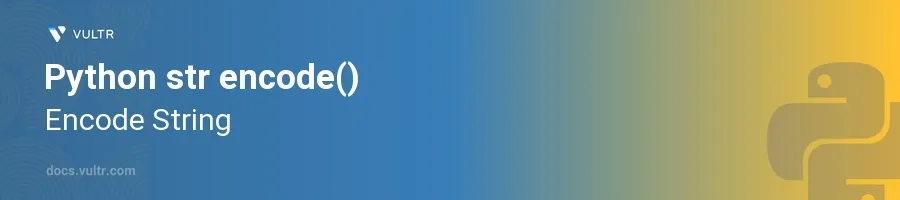
Introduction
The encode()
method in Python is a vital tool when you need to convert a string from the default Unicode encoding to a specific encoded version. This methodology is particularly crucial in data processing where encoding consistency is necessary across different systems, or when interacting with network resources or file systems that expect data in a specific encoding format.
In this article, you will learn how to efficiently use the encode()
method on strings in Python. You will discover various applications of this method, understand common encodings, and see practical examples demonstrating how to encode strings using different character sets.
Basic Encoding with encode()
Encode to UTF-8
Start with a basic string in Python.
Use the
encode()
function to convert it into UTF-8 format.pythonoriginal_string = "Python programming is fun!" encoded_string = original_string.encode('utf-8') print(encoded_string)
The output will be displayed in byte format, indicating that the string has been successfully converted to UTF-8 encoding.
Encode to ASCII
Define a simple ASCII compatible string.
Apply the
encode()
function specifying ASCII as the target encoding.pythonsimple_string = "Data Science 101" ascii_encoded = simple_string.encode('ascii') print(ascii_encoded)
This will encode the string using ASCII. Non-ASCII characters, when encountered, will raise an error unless explicitly handled.
Handling Non-ASCII Characters
Encode with Errors Handling
When encoding non-ASCII characters, handling errors efficiently is essential. Python's encode()
method offers multiple strategies to deal with characters that do not fall within the specified encoding.
Choose a string with non-ASCII characters.
Encode this string and handle errors using different strategies like 'ignore', 'replace', or 'xmlcharrefreplace'.
pythonnon_ascii_string = "Résumé for café" encoded_ignore = non_ascii_string.encode('ascii', 'ignore') encoded_replace = non_ascii_string.encode('ascii', 'replace') encoded_xmlreplace = non_ascii_string.encode('ascii', 'xmlcharrefreplace') print("Ignore Errors: ", encoded_ignore) print("Replace Errors: ", encoded_replace) print("XML Char Refs: ", encoded_xmlreplace)
- 'ignore' skips characters that are not representable in ASCII.
- 'replace' replaces non-representable characters with '?'.
- 'xmlcharrefreplace' replaces non-representable characters with XML character references.
Explore Other Encodings
Encoding with ISO-8859-1
Latin-1 or ISO-8859-1 is a single-byte encoding that can represent the first 256 Unicode characters. It's a common encoding for data from older systems and Western European languages.
Select a string that contains characters within the ISO-8859-1 character set.
Encode the string using ISO-8859-1 without needing special error handling.
pythoneuro_string = "Euro symbol: €" encoded_latin1 = euro_string.encode('iso-8859-1') print(encoded_latin1)
This method smoothly handles characters present in the Latin-1 character set including the Euro symbol.
Conclusion
By mastering the encode()
function in Python, effectively manage string encoding for various applications, whether it's for file I/O, network communication, or data processing across different locales and systems. Remember, choosing the correct encoding and error handling strategy is crucial depending on the data's language and character set. Implement these encoding techniques to ensure your applications are robust and data is consistent across different systems.
No comments yet.