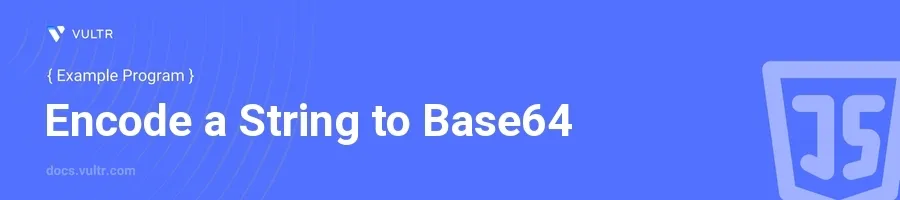
Introduction
Base64 encoding is a widely-used method to represent binary data in ASCII string format, primarily used in scenarios where data needs to be stored and transferred over media designed to deal with textual data. This ensures that the data remains intact without modification during transport. Base64 is commonly used in various programming environments, including web development, to encode and embed binary data within scripts, data files, and HTML pages.
In this article, you will learn how to encode a string to Base64 using JavaScript. A step-by-step approach will guide you through using both built-in methods provided by JavaScript and manually implementing the encoding process. This will provide a solid understanding of how Base64 encoding works and how it can be implemented in JavaScript programs.
Using Built-in JavaScript Functions to Encode Base64
JavaScript provides built-in functions such as btoa()
to handle Base64 encoding directly. Here’s how you use this function to convert a string into a Base64-encoded format:
Encode a Simple String
Declare the string you want to encode.
Use the
btoa()
function to encode the string.javascriptconst originalString = "Hello, World!"; const encodedString = btoa(originalString); console.log(encodedString);
This snippet takes the string "Hello, World!" and encodes it to Base64. The result is output to the console.
Considerations with Unicode Characters
Note that
btoa()
does not directly support characters that are outside the Latin1 range.To handle Unicode strings, encode them properly before converting to Base64.
javascriptconst unicodeString = "Привет, мир!"; const encodedUnicodeString = btoa(unescape(encodeURIComponent(unicodeString))); console.log(encodedUnicodeString);
This code first uses
encodeURIComponent()
to escape the string, making it ASCII-safe.unescape()
is then used to ensure the string is converted back into a character string for Base64 encoding.
Manually Implementing Base64 Encoding
While the built-in functions are handy, understanding the underlying process of Base64 encoding can be beneficial. Here’s how you can implement your own Base64 encoding function in JavaScript:
Create a Base64 Character Set
Define all characters used in Base64 encoding.
javascriptconst BASE64_CHARS = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/";
Encode a String to Base64 Manually
Convert each character of the input string into binary.
Group the binary bits into sets of 6 since Base64 represents each character as 6 bits.
Map these sets of 6 bits to the corresponding Base64 character.
javascriptfunction encodeToBase64(input) { let output = ""; let asciiValue, binaryForm, base64Chunk; for (let i = 0; i < input.length; i++) { asciiValue = input.charCodeAt(i); binaryForm = asciiValue.toString(2).padStart(8, '0'); base64Chunk = parseInt(binaryForm, 2); output += BASE64_CHARS[base64Chunk >> 2]; } return output; } console.log(encodeToBase64("Hello, World!"));
This function converts an input string to its corresponding Base64 encoded form by following encoding rules step-by-step and mapping each calculated binary form to the Base64 charset.
Conclusion
Encoding strings into Base64 in JavaScript is straightforward with the built-in btoa()
function, while understanding the encoding manually promotes deeper insights into data handling within web applications. Remember that the btoa()
function is not fully Unicode-compliant out of the box and requires encoding strings properly before conversion. Whether using built-in methods or implementing the Base64 encoding yourself, JavaScript offers comprehensive options for managing encoded data effectively.
No comments yet.