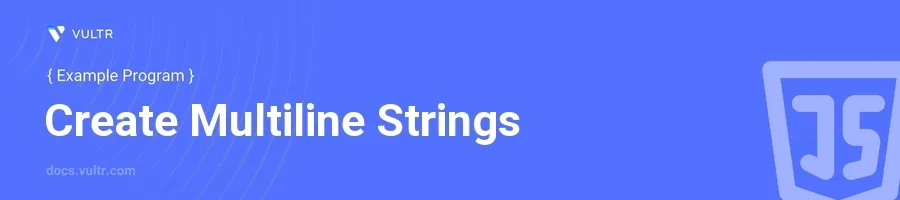
Introduction
Multiline strings in JavaScript can greatly simplify the management of long text blocks within your code. Whether you're working with large chunks of HTML, complex SQL queries, or simply long messages, managing multiline strings effectively can lead to cleaner, more readable code. Traditionally, JavaScript handled multiline strings less conveniently, but modern syntax introduced in ES6 has greatly improved this aspect.
In this article, you will learn how to create and manage multiline strings in JavaScript through several examples. Discover techniques using both older JavaScript practices and the newer, more efficient template literals introduced in ES6.
Traditional JavaScript Methods for Multiline Strings
Using the Plus Operator
Concatenate each line using the plus (
+
) operator.Ensure that each line is a properly quoted string and ends with a space if needed.
javascriptvar multilineString = "This is a string " + "that spans across " + "multiple lines."; console.log(multilineString);
This method manually creates a multiline string by adding each line to the next with the plus operator. The code effectively forms a long sentence that is broken into separate strings for readability.
Using the Backslash
End each line with a backslash (
\
) to indicate continuation.Avoid adding any characters (including spaces or tabs) after the backslash, as it may cause syntax errors.
Preserve the indentation for readability without affecting the string content.
javascriptvar multilineString = "This is another example \ of a multiline string \ in JavaScript."; console.log(multilineString);
Here, the backslash allows the string to continue to the next line in the source code, but it doesn’t include line breaks in the actual string output.
ES6 Template Literals for Multiline Strings
Simple Multiline String
Use backticks (
`
) to wrap the multiline string.Simply press "Enter" where a line break is needed in the string.
javascriptlet multilineString = `This is a string that spans across multiple lines using ES6 syntax.`; console.log(multilineString);
The template literals allow actual line breaks in the code, which directly translate into the string value, thereby simplifying the syntax and improving readability.
Interpolating Variables and Expressions
Include variables and expressions using
${expression}
syntax within template literals.Combine text and variables seamlessly over multiple lines.
javascriptlet name = 'world'; let multilineString = `Hello, ${name}! Today is ${new Date().toDateString()}. Enjoy coding!`; console.log(multilineString);
Template literals not only support multiline strings but also embedding expressions, which includes variables, function calls, and calculations. This enhances flexibility when constructing strings.
Handling Indentation
Write the entire string aligned to the left edge to avoid unwanted spaces in the result.
Use a function to strip the initial whitespace if alignment in the code is necessary for readability.
javascriptfunction stripIndent(str) { const match = str.match(/^[ \t]*(?=\S)/gm); if (!match) return str; const indent = Math.min(...match.map(el => el.length)); const re = new RegExp(`^[ \\t]{${indent}}`, 'gm'); return str.replace(re, ''); } let multilineString = stripIndent(` This is a formatted string, neatly aligned in the code, but without leading spaces.`); console.log(multilineString);
This function detects the smallest indent for all lines and removes it, making it possible to maintain a clean and readable alignment in the code without affecting the output.
Conclusion
Creating multiline strings in JavaScript is flexible and intuitive, especially with the use of ES6 template literals. Utilize these methods to simplify the handling of long strings in your JavaScript code, thus making your scripts more maintainable and elegant. Ensure to choose the right approach based on your project's needs to maintain readability and performance in your development practices.
No comments yet.