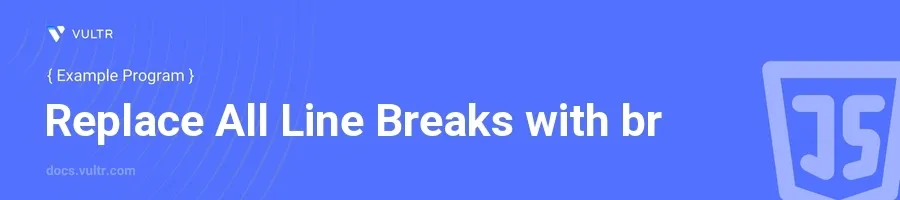
Introduction
Handling text formatting in web applications is a common task, and replacing line breaks with <br>
tags in JavaScript is essential for displaying multiline text correctly in HTML. This operation is useful when you have text input that includes line breaks for readability but needs to be displayed within a HTML page, which does not recognize raw line breaks.
In this article, you will learn how to replace all line breaks in a string with <br>
tags using JavaScript. The examples provided will demonstrate various methods to achieve this, including using regular expressions and the replace
method.
Replacing Line Breaks with <br>
Tags
Basic Replacement Using a String
The simplest way to replace line breaks with <br>
tags is to use the String.prototype.replace()
function with a string that represents a line break.
Define a string that includes line breaks using escape characters.
Utilize the
replace()
function with'\n'
as the target and'<br>'
as the replacement.javascriptvar text = "Hello,\nHow are you?\nI am fine."; var newText = text.replace('\n', '<br>'); console.log(newText);
In this example, only the first occurrence of the line break is replaced because the
replace()
method, when used with a string as the search term, only replaces the first match.
Using Global Regular Expression
To replace all line breaks in a string, use a regular expression with the global flag (/g
).
Follow the same initial steps to define a string with line breaks.
Apply the
replace()
function with a regular expression that matches all line breaks.javascriptvar text = "Hello,\nHow are you?\nI am fine."; var newText = text.replace(/\n/g, '<br>'); console.log(newText);
This snippet ensures that all line breaks in
text
are replaced with<br>
. The/g
flag in the regular expression is crucial for global replacement.
Handling Different Types of Line Breaks
Different operating systems and environments might use various characters for line breaks, such as \r\n
for Windows or \r
for older Macintosh systems. You need a robust solution for all environments.
Consider all common line break types in the regular expression.
Replace each type of line break with
<br>
, ensuring compatibility across platforms.javascriptvar text = "Hello,\r\nHow are you?\rI am fine."; var newText = text.replace(/(\r\n|\r|\n)/g, '<br>'); console.log(newText);
This regular expression matches all types of line breaks (
\r\n
,\r
, and\n
) and replaces them with<br>
. It covers the variations you might encounter across different text sources and platforms.
Conclusion
Replacing line breaks with <br>
tags is a straightforward yet powerful method in JavaScript to prepare text for HTML display. Whether dealing with browser inputs or string literals in your JavaScript code, understanding how to manipulate line breaks is essential for ensuring correct formatting. Utilize these methods to handle and display multiline strings elegantly within your web applications. By mastering these techniques, you ensure that text formatting in your JavaScript applications is both versatile and robust.
No comments yet.