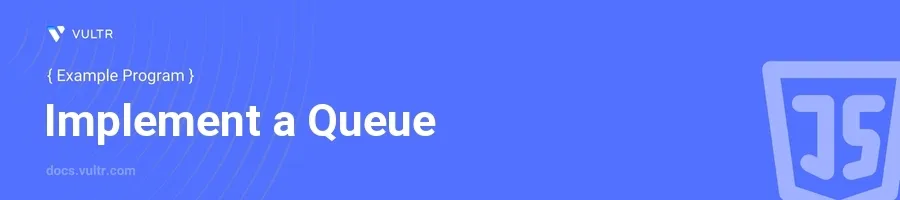
Introduction
A queue is a fundamental data structure in computer science that operates under the principle of First In, First Out (FIFO). This means that the first element added to the queue will be the first one to be removed. JavaScript, being a versatile language, allows the implementation of queues using arrays or custom classes, which is especially useful in scenarios like handling tasks in a specific order, managing data buffers, or in breadth-first search algorithms.
In this article, you will learn how to implement a queue in JavaScript through practical examples. Discover how to manage a queue using JavaScript's array methods as well as how to create a more robust queue structure by defining a custom Queue class. These concepts will enhance your understanding of data structures and their usage in JavaScript programming.
Implementing Queue Using JavaScript Arrays
Simple JavaScript Queue Operations
Understand the array methods that can simulate queue operations.
Use
push()
to add elements to the end of the queue.Use
shift()
to remove elements from the front of the queue.javascriptlet queue = []; queue.push('Apple'); queue.push('Banana'); console.log(queue.shift()); // Outputs: Apple console.log(queue.shift()); // Outputs: Banana
This example initializes an array
queue
and usespush()
to add elements.shift()
is then used to remove and output elements in the order they were added, demonstrating the FIFO nature of a JavaScript queue.
Checking If Queue Is Empty
Verify the length of the array to determine if the queue is empty.
Return a boolean value based on the queue's length.
javascriptfunction isQueueEmpty(queue) { return queue.length === 0; } let myQueue = ['Apple', 'Banana']; console.log(isQueueEmpty(myQueue)); // Outputs: False myQueue.shift(); myQueue.shift(); console.log(isQueueEmpty(myQueue)); // Outputs: True
In this snippet, the
isQueueEmpty
function checks if the queue is empty by comparing its length to0
. This helps in managing queue operations without causing any errors when attempting to remove an element from an empty queue in js.
Implementing Queue Using JavaScript Class
Defining the Queue Class
Start by creating a Queue class with an empty list to store our elements.
Write
enqueue(element)
method for adding elements.Write
dequeue()
method for removing elements.Add a method to check if the queue is empty.
javascriptclass Queue { constructor() { this.items = []; } enqueue(item) { this.items.push(item); } dequeue() { if (this.isEmpty()) { console.log("Queue is empty!"); return null; } return this.items.shift(); } isEmpty() { return this.items.length === 0; } }
Here, the
Queue
class is defined with methods for enqueueing (adding to the queue), dequeueing (removing from the queue), and checking if the queue is empty. Thedequeue
method first checks if the queue is empty to prevent removing an element from an empty queue.
Using the Queue Class
Initialize an instance of the Queue class.
Perform enqueue and dequeue operations to see it in action.
javascriptlet myQueue = new Queue(); myQueue.enqueue('Red'); myQueue.enqueue('Blue'); console.log(myQueue.dequeue()); // Outputs: Red console.log(myQueue.dequeue()); // Outputs: Blue console.log(myQueue.dequeue()); // Outputs: Queue is empty! and returns null
In this code segment, an instance of the Queue class is created and used to add and remove elements. The FIFO behavior is maintained, and an appropriate message is displayed when trying to dequeue from an empty queue.
Conclusion
Implementing a queue in JavaScript can be accomplished using either array methods or by defining a custom Queue class. Utilizing arrays provides a straightforward approach suitable for simple scenarios, whereas a custom class offers greater control and encapsulation, making it fitting for more complex applications. Employ these techniques to effectively manage data in your JavaScript applications, ensuring that data handling is structured and predictable, especially in scenarios that crucially depend on the order of execution.
No comments yet.