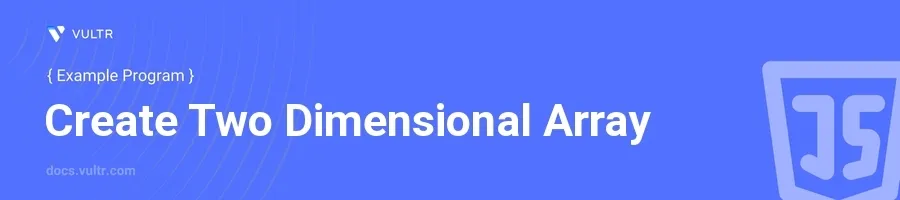
Introduction
A two-dimensional array in JavaScript is essentially an array of arrays, where each element of the main array holds another array. This concept is vital in handling grouped data, such as matrices or grids, where you might need to store values in rows and columns. JavaScript doesn't support true multidimensional arrays like some other languages, but the same functionality can be achieved using arrays of arrays.
In this article, you will learn how to create and manipulate two-dimensional arrays in JavaScript through detailed examples. Master techniques to initialize, access, and modify the data within these structures effectively.
Creating Two Dimensional Arrays
Initializing a Two Dimensional Array
Declare an empty two-dimensional array.
Populate the array with nested arrays representing the rows.
javascriptvar twoDArray = []; twoDArray[0] = [1, 2, 3]; twoDArray[1] = [4, 5, 6]; twoDArray[2] = [7, 8, 9];
This code sets up a
2D
array with three rows and three columns. Each row is an array that is assigned to an index of the maintwoDArray
.
Using Array Literals
Define the two-dimensional array using array literals directly.
This method provides a concise way to create pre-filled arrays.
javascriptvar twoDArray = [ [1, 2, 3], [4, 5, 6], [7, 8, 9] ];
Here,
twoDArray
is initialized with three rows filled with numbers from 1 to 9. Array literals simplify initialization and make the code easier to read.
Creating a Two Dimensional Array Dynamically
Use loops to generate both dimensions of the array.
This approach is useful when the array dimensions are determined at runtime.
javascriptvar rows = 4; var cols = 5; var dynamicArray = []; for(var i = 0; i < rows; i++) { dynamicArray[i] = []; for(var j = 0; j < cols; j++) { dynamicArray[i][j] = j + 1; } }
The above snippet dynamically creates a two-dimensional array with 4 rows and 5 columns, where each column entry is filled sequentially from 1 to 5.
Manipulating Data in Two Dimensional Arrays
Accessing Elements
Access elements using their row and column indices.
It’s crucial to navigate through the layers of the array accurately.
javascriptvar value = twoDArray[1][2]; // Accesses the third element of the second row console.log(value); // Outputs: 6
This code retrieves the value '6' from the two-dimensional array, which is in the second row and third column.
Modifying Elements
Modify individual elements by targeting them through their indices.
Remember that array indices start at '0'.
javascripttwoDArray[0][0] = 10; // Changes the first element of the first row to 10 console.log(twoDArray[0][0]); // Outputs: 10
Here, the first element of the first row is changed to '10'. The array reflects this change, showing the flexibility of two-dimensional arrays in storing and modifying data.
Conclusion
Two-dimensional arrays in JavaScript are an essential tool for handling data in a structured format similar to matrices. They provide the ability to store rows and columns of data efficiently. By using nested arrays, you can mimic the functionality of multidimensional arrays, which are crucial in applications ranging from data processing to graphics and gaming. With these examples, take full advantage of JavaScript arrays to manage complex data structures effectively. From creating empty or pre-filled arrays to manipulating their content dynamically, employ these techniques to enhance your programming projects.
No comments yet.