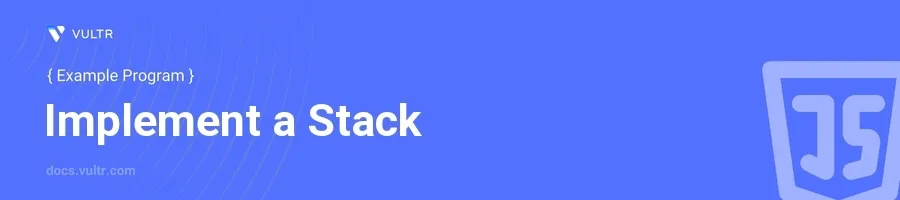
Introduction
A Stack is a fundamental data structure in computer science, serving as a collection of elements with two principal operations: push, which adds an element to the collection, and pop, which removes the most recently added element. JavaScript does not include a built-in stack data structure, but implementing one is crucial for understanding concepts such as function calls, parsing expressions, iterating data, and more.
In this article, you will learn how to implement a stack using JavaScript. Discover how to define the Stack class, incorporate essential methods like push, pop, peek, and isEmpty, and learn to use your stack through practical examples.
Implementing Stack in JavaScript
Defining the Stack Class
The basis of a stack implementation in JavaScript is creating a class that encapsulates the stack's properties and behaviors.
Define the Stack class.
Initialize an empty array to store stack items.
javascriptclass Stack { constructor() { this.items = []; } }
Here, a class named
Stack
is declared with a constructor that initializes an empty arrayitems
to hold the stack elements.
Adding Elements with Push
The push method adds an element to the top of the stack.
Implement the
push
method inside the Stack class.Add an element to the stack array.
javascriptpush(element) { this.items.push(element); }
This code snippet defines the
push
method, which takes anelement
as a parameter and uses the array'spush
method to add it to theitems
array.
Removing Elements with Pop
The pop method removes the element at the top of the stack.
Implement the
pop
method.Ensure the stack isn't empty.
Return the popped element.
javascriptpop() { if (this.isEmpty()) { return 'Stack is empty'; } return this.items.pop(); }
This method first checks if the stack is empty using the
isEmpty
method. If not, it removes the top element using the array'spop
method.
Peek at the Top Element
The peek method returns the top element from the stack without removing it.
Implement the
peek
method.Check if the stack is empty.
Return the top element.
javascriptpeek() { if (this.isEmpty()) { return 'Stack is empty'; } return this.items[this.items.length - 1]; }
peek
checks if the stack is empty. If not, it returns the top element without removing it.
Check if Stack is Empty
Determining whether the stack is empty is frequently required before operations like pop or peek.
Implement the
isEmpty
method.Return a boolean indicating if the stack is empty.
javascriptisEmpty() { return this.items.length === 0; }
This method returns
true
if theitems
array is empty, otherwisefalse
.
Using the Stack Class
After establishing the Stack class with its corresponding methods, use the stack to demonstrate its functionality.
Create an instance of the Stack class.
Push elements onto the stack.
Peek at the top element.
Pop elements and display them.
javascriptconst stack = new Stack(); stack.push(10); stack.push(20); stack.push(30); console.log(stack.peek()); // Output: 30 console.log(stack.pop()); // Output: 30 console.log(stack.pop()); // Output: 20 console.log(stack.pop()); // Output: 10
In this example, a new stack is created, several integers are pushed onto it, and the top element is checked and removed to demonstrate the stack's LIFO (last-in-first-out) behavior.
Conclusion
Implementing a stack in JavaScript enhances understanding of fundamental programming concepts and data structure management. Through the discussed methods like push, pop, peek, and isEmpty within a custom Stack class, you gain the ability to manage elements in a controlled, LIFO manner. Utilize these techniques to handle data where the order of insertion and removal is crucial for your application's logic. With the steps covered, you ensure a robust, practical understanding of using stacks in JavaScript programming.
No comments yet.