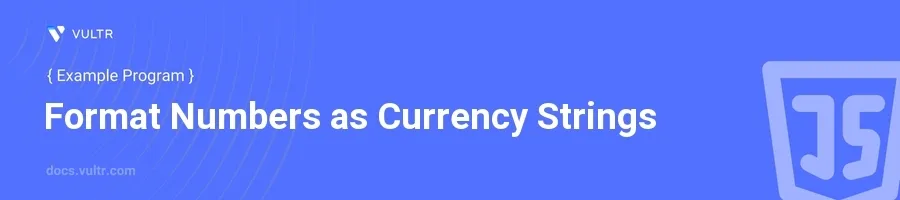
Introduction
Formatting numbers as currency strings is a common task in many applications, whether you're building an e-commerce site, a financial dashboard, or any system that handles monetary values. JavaScript provides straightforward ways to handle this, ensuring that numeric data is presented to users in a readable and localized format.
In this article, you will learn how to leverage JavaScript to transform numerical values into well-formatted currency strings. Explore various methods, including the use of the Intl.NumberFormat
object and toLocaleString method to achieve localization in your applications.
Basic Currency Formatting in JavaScript
Using toLocaleString
Recognize the availability of the
toLocaleString
method for numbers.Format a number as a currency string specifying the locale and currency options.
javascriptlet amount = 123456.789; let formatted = amount.toLocaleString('en-US', { style: 'currency', currency: 'USD' }); console.log(formatted);
Here,
toLocaleString()
is used to formatamount
as a currency string in US English locale with the US Dollar as the currency. The output will be$123,456.79
, displaying the number in a typical monetary format suitable for financial displays.
Customizing the Display of Currency
Adjust the number of decimal places in the output.
Use
maximumFractionDigits
andminimumFractionDigits
to set precision.javascriptlet amount = 1000; let formatted = amount.toLocaleString('en-US', { style: 'currency', currency: 'USD', minimumFractionDigits: 0, maximumFractionDigits: 0 }); console.log(formatted);
This code formats the
amount
with no decimal places, displaying$1,000
. It's useful for rounded currency amounts where cents are not needed.
Advanced Currency Formatting with Intl.NumberFormat
Initializing Intl.NumberFormat
Create an
Intl.NumberFormat
object for repeated use.Specify the desired locale and currency options during initialization.
javascriptconst formatter = new Intl.NumberFormat('en-GB', { style: 'currency', currency: 'GBP' });
This initializes a formatter for British Pound Sterling, with settings appropriate for the UK locale. Use this formatter multiple times throughout the application for consistent formatting.
Formatting Multiple Numbers
Use the
formatter
object created earlier to format various numbers.Apply the
format
method of theIntl.NumberFormat
object on each number.javascriptlet prices = [99.99, 2500, 150.75]; let formattedPrices = prices.map(price => formatter.format(price)); console.log(formattedPrices);
This code snippet formats an array of numbers into GBP currency strings. The resulting array
formattedPrices
contains values like£99.99
,£2,500.00
, and£150.75
.
Practical Usage in Web Development
Integrating Currency Formatting into HTML
Handle user input for a price.
Format the inputted value as soon as it's entered.
javascriptdocument.getElementById("priceInput").addEventListener("change", function(event) { let rawValue = parseFloat(event.target.value); let formattedValue = rawValue.toLocaleString('en-US', { style: 'currency', currency: 'USD' }); document.getElementById("formattedPrice").textContent = formattedValue; });
This JavaScript code listens for changes in an input field (
priceInput
), formats the entered value as a US Dollar string, and immediately displays the formatted value in another HTML element (formattedPrice
).
Dynamic Currency Formatting Based on User Selection
Allow users to select their preferred currency.
Format numbers based on the selected currency dynamically.
javascriptfunction updateCurrency(value, currency) { return value.toLocaleString('en-US', { style: 'currency', currency: currency }); } // Assume the user selects 'EUR' let userCurrency = 'EUR'; let productPrice = 350; console.log(updateCurrency(productPrice, userCurrency));
The
updateCurrency
function shown here takes a numerical value and a currency code, returning a string formatted accordingly. This allows flexibility in applications serving a global audience, where users might prefer to see prices in their local currency.
Conclusion
Mastering the generation of currency strings in JavaScript enhances the user interface of any application dealing with financial transactions. By using the built-in JavaScript functions like toLocaleString
and Intl.NumberFormat
, you easily present numbers in more accessible, localized formats. Implement these methods to improve data readability, adapt to user preferences, and ensure consistent presentation of monetary values across different parts of your application.
No comments yet.