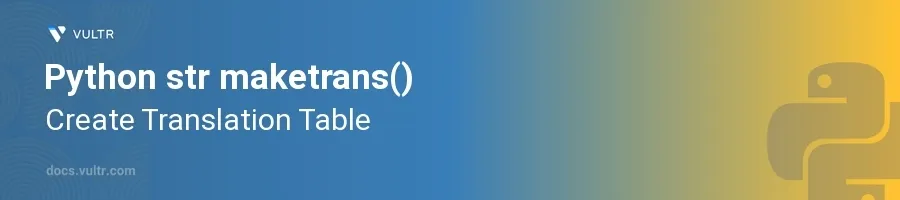
Introduction
The maketrans()
method in Python is a static method of the str
class that creates a translation table, which is a mapping of integers or characters to integers, strings, or None. This translation table can then be used with Python's translate()
method to modify strings. The maketrans()
method is particularly useful for data cleaning, preprocessing in machine learning, or anywhere where string manipulation is necessary.
In this article, you will learn how to create and utilize translation tables using the maketrans()
and translate()
methods in Python. Discover how to replace, remove, or modify characters in strings effectively through practical examples and step-by-step guidance.
Understanding str maketrans()
Syntax and Parameters
Examine the typical syntax of the
maketrans()
method:pythonstr.maketrans(x[, y[, z]])
Understand the parameters:
x
can be a dictionary describing a one-to-one mapping of Unicode ordinals or characters to Unicode ordinals, strings, orNone
.y
is optional and represents a string of characters to map to the string of equal lengthz
.z
is optional and represents a string where each character in the string is mapped to None in the translation table.
Types of Input for maketrans()
Using a Dictionary: Define a dictionary where each key-value pair represents the character to be replaced and the character to replace it with.
pythontranslate_dict = {'a': '1', 'e': '2'} trans_table = str.maketrans(translate_dict)
Using two Strings: Define two strings of equal length where characters in the first string are replaced by characters in the same position in the second string.
pythontrans_table = str.maketrans('aeiou', '12345')
Using three Strings (to remove characters): Define a third string where each character in this string will be mapped to None.
pythontrans_table = str.maketrans('aeiou', '12345', 'xyz')
Applications of Translation Tables
- Character Replacement: Change specific characters to other characters.
- Data Cleaning: Remove unwanted characters from strings.
- Character Mapping: Standardize character sets in text processing tasks.
Implementing maketrans() and translate()
Basic Translation and Replacement
Create a basic translation table.
pythontrans_table = str.maketrans('abc', '123')
Use the translation table with
translate()
to modify a string.pythonsample_string = 'Hello, beautiful world and amazing people!' result = sample_string.translate(trans_table) print(result)
This will replace 'a' with '1', 'b' with '2', and 'c' with '3' in the
sample_string
.
Removing Characters
Create a translation table that maps characters to None.
pythontrans_table = str.maketrans('', '', 'aeiou')
Apply the translation table to remove all vowels from a string.
pythonsample_string = "Live, Learn, and Thrive!" result = sample_string.translate(trans_table) print(result)
This results in "Lv, Lrn, nd Thrve!", having all vowels removed.
Conclusion
The str.maketrans()
method in Python offers a powerful way to create translation tables that efficiently modify strings using the translate()
method. By following the examples and explanations provided, master replacing, removing, or altering characters in strings for various applications like data cleaning or preprocessing. These operations significantly enhance string manipulation capabilities, making your text processing tasks more efficient and streamlined. Harness the potential of maketrans()
and translate()
to make your Python scripting more effective and tailored to your needs.
No comments yet.