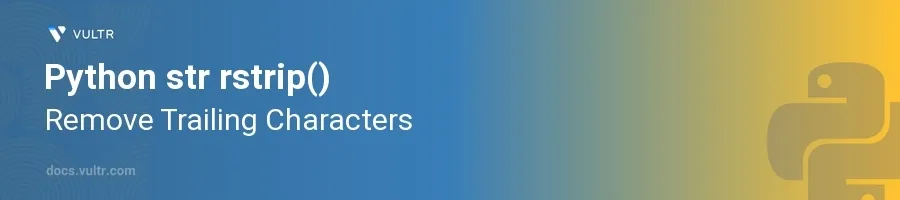
Introduction
The rstrip()
method in Python is a string method used to remove trailing characters (characters at the end of a string). This method is particularly useful for cleaning up strings, especially in data processing where you might need to remove unnecessary whitespace or specific unwanted characters from the end of strings before further processing.
In this article, you will learn how to use the rstrip()
method to effectively remove trailing characters from strings in Python. Discover how to apply this method not only for removing spaces but also for stripping away specific sets of characters, enhancing the manageability and presentation of string data.
Basic Usage of rstrip()
Removing Trailing Whitespaces
Start with a basic string that contains trailing spaces.
Use the
rstrip()
method to remove the spaces.pythonexample_string = "Hello World! " trimmed_string = example_string.rstrip() print(f"Original: '{example_string}'") print(f"Trimmed: '{trimmed_string}'")
The
rstrip()
method, when called without arguments, defaults to removing any whitespace characters (spaces, tabs, newlines) at the end of the string. In this example, it removes the spaces after "Hello World!".
Handling Tabs and Newlines
Create a string that ends with a newline and tabs.
Apply the
rstrip()
method to clean up the string.pythonmessy_string = "Data Entry\t\t\n" clean_string = messy_string.rstrip() print(f"Cleaned: '{clean_string}'")
This code snippet shows
rstrip()
removing not just spaces but also tab (\t
) and newline (\n
) characters from the end of the string.
Stripping Specific Characters
Removing Specific Trailing Characters
Define a string with specific characters at the end that you want to remove.
Pass a string specifying these characters to the
rstrip()
method.pythonurl = "www.example.com/////" clean_url = url.rstrip('/') print(f"Clean URL: '{clean_url}'")
In this example,
rstrip()
is used to remove all trailing slashes from a URL.
Complex Character Sets
Consider a scenario with trailing punctuation and special characters.
Use the
rstrip()
method with a combination of characters.pythonfilename = "document.txt!!!!???" clean_filename = filename.rstrip('!?') print(f"Filename: '{clean_filename}'")
This snippet demonstrates the removal of multiple different trailing characters ('!', '?') in one operation. The order of characters in the argument does not affect the result.
Practical Applications
Cleaning Up User Input
Strip unwanted characters from user input to prevent common errors or misuse.
pythonuser_input = input("Enter your name: ").rstrip() print(f"Hello, {user_input}!")
Utilizing
rstrip()
to clean up user input helps in maintaining consistency and preventing issues related to unexpected extra trailing whitespaces.
Preparing Data for Storage or Processing
Remove trailing characters from data before saving to a database or further processing.
pythondata = "some important info " processed_data = data.rstrip() # Save or process data
Removing trailing spaces or other specified characters ensures that the data stored or processed is clean and exactly as required.
Conclusion
The rstrip()
function in Python is an invaluable tool for string manipulation, enabling the removal of unwanted trailing characters with ease. By understanding and using rstrip()
in different scenarios—from cleaning data to user inputs—you empower your programs to handle string data more effectively and reliably. With clear examples and practical applications outlined, start integrating rstrip()
in your Python projects to maintain clean and effective string data handling.
No comments yet.