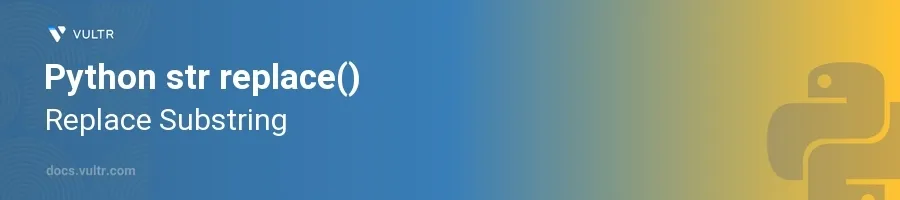
Introduction
The replace()
method in Python strings is a versatile tool for substituting parts of the string with new content. This method is commonly used for cleaning text data, creating modified copies of strings, or developing user-friendly outputs without complex loops or conditional statements. Knowing how to efficiently use replace()
can significantly streamline various tasks involving text manipulation.
In this article, you will learn how to use the replace()
method effectively within different contexts. Dive into scenarios including basic text replacement, case-sensitive modifications, and using replace()
within more extensive data processing tasks.
Basic Usage of replace()
Replace a Simple Substring
Start with a basic string.
Use the
replace()
to substitute a specified substring with a new one.pythonoriginal = "Hello World!" modified = original.replace("World", "Universe") print(modified)
This snippet replaces "World" with "Universe". The output will be
Hello Universe!
.
Handling Non-Existing Substrings
Understand that if the substring does not exist,
replace()
simply returns the original string.Try replacing a substring that doesn't exist in the original string.
pythonmodified = original.replace("Goodbye", "Hello") print(modified)
Since "Goodbye" is not found, the output remains unchanged as
Hello World!
.
Advanced Text Manipulation
Case-Sensitive Replacements
Recognize that
replace()
is case-sensitive.Perform a case-sensitive modification.
pythoncase_text = "Apple and apple are different." modified_case_text = case_text.replace("apple", "orange") print(modified_case_text)
This code replaces only the lowercase "apple" with "orange", resulting in the string
Apple and orange are different.
.
Limiting the Number of Replacements
Sometimes, controlling the number of substitutions is necessary.
Use the optional third argument of
replace()
to limit replacements.pythonrepeated_text = "apple apple apple" modified_limited = repeated_text.replace("apple", "orange", 2) print(modified_limited)
Here, only the first two "apple" substrings are replaced by "orange", giving
orange orange apple
.
Using replace() in Data Processing
Cleaning Up Text Data
Use
replace()
to remove unnecessary or unwanted characters.Apply it to a more complex string cleanup task.
pythonmessy_data = "Name: John; Age: 30; City: New York;" clean_data = messy_data.replace(";", "") print(clean_data)
This code removes all semicolons, simplifying further parsing or data processing, resulting in
Name: John Age: 30 City: New York
.
Chain Replacements for Multiple Adjustments
Chain multiple
replace()
methods to perform several replacements in a single statement.Address several issues in one line of code.
pythondeeply_messy_data = "Name: John; Age: 30; City: New York; [Delete]" cleaned_data = deeply_messy_data.replace(";", "").replace("[Delete]", "") print(cleaned_data)
This cleans up the data by removing both semicolons and the "[Delete]" string, resulting in a more usable format
Name: John Age: 30 City: New York
.
Conclusion
The replace()
function in Python provides a powerful yet simple means for manipulating strings by replacing substrings efficiently. Whether dealing with basic text replacements or complex data cleaning operations, replace()
offers a direct approach for modifying Python strings. By mastering string replacements and understanding how to apply them in varied scenarios, enhance text processing tasks and improve the automation and quality of data cleaning workflows.
No comments yet.