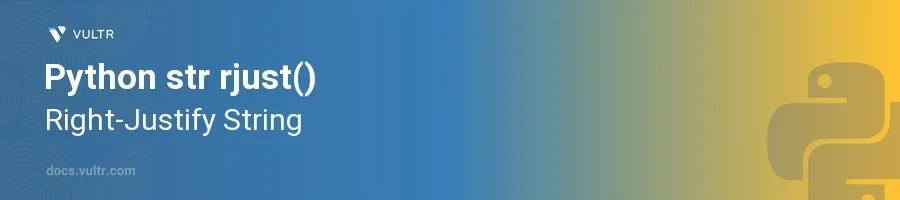
Introduction
The str.rjust()
method in Python is a built-in String method used for right-justifying string data. This function is typically used to align text to the right side of a specified width by padding it on the left with a specified fill character or the default space character. Understanding how to use rjust()
can be particularly useful in creating output that requires a certain format, such as reports or tables.
In this article, you will learn how to effectively use the str.rjust()
method in Python for various applications. Discover how to right-justify strings, customize padding, and apply this method to practical programming scenarios such as formatting tables or aligning text output.
Basic Usage of rjust()
Right-Justifying a String
Take a string that you need to right-justify.
Determine the total width for the justification.
Call the
rjust()
method on the string.pythontext = "Hello" justified_text = text.rjust(10) print(justified_text)
This code snippet right-justifies the string
"Hello"
to a width of 10 characters. The output will show spaces padded to the left:" Hello"
.
Using Custom Padding Character
Choose a string and the width for right-justification.
Specify a character for padding instead of the default space.
Apply the
rjust()
method using the custom character.pythontext = "Hello" justified_text = text.rjust(10, '-') print(justified_text)
Here, the
rjust()
method pads the string"Hello"
with dashes on the left, producing the output:"-----Hello"
.
Practical Applications of rjust()
Aligning Columns in Text Output
Prepare a list of strings of varying lengths.
Specify a column width that is at least as wide as the longest string.
Right-justify each string in the list using
rjust()
.pythonnames = ["Alice", "Bob", "Christine"] max_length = max(len(name) for name in names) for name in names: print(name.rjust(max_length))
This process iterates over the names, right-justifying each one to the maximum length of the names in the list, ensuring each is aligned properly on the right.
Formatting Tables
Define the data and headers for a table.
Decide on the column widths based on the longest item in each column.
Use
rjust()
for each item while assembling the rows.pythonheaders = ["ID", "Name", "Age"] data = [ [1, "Alice", 30], [2, "Bob", 22], [3, "Christine", 45] ] col_widths = [max(len(str(row[i])) for row in data) for i in range(len(headers))] print(" | ".join(header.rjust(col_widths[i]) for i, header in enumerate(headers))) for row in data: print(" | ".join(str(item).rjust(col_widths[i]) for i, item in enumerate(row)))
This procedure aligns each column's headers and data entries to the right, ensuring all elements are vertically aligned and properly formatted.
Conclusion
The str.rjust()
method in Python simplifies the process of aligning text to the right, a common requirement for various text formatting tasks. Whether you're working on generating reports, creating formatted text output, or designing well-structured tables, mastering rjust()
enhances your ability to deliver clear and organized text presentations. By implementing the techniques discussed, enhance the readability and professionalism of your Python scripts.
No comments yet.