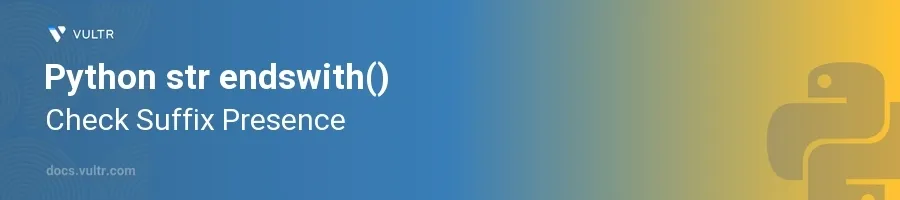
Introduction
The endswith()
method in Python is a string method used to check if a string ends with a specified suffix. This method is particularly useful in parsing and data validation situations where determining the tail of a text string is needed, such as file extensions or certain coding conventions.
In this article, you will learn how to effectively use the endswith()
method to ensure text meets certain ending criteria. You will explore various examples that demonstrate checking suffixes in simple strings, within conditions, and across multiple options.
Understanding str.endswith()
Basic Usage of endswith()
The endswith()
method requires at least one parameter — the suffix you want to check for. Optionally, it can take additional parameters specifying a range to check within the string.
Define a string you want to check.
Apply
endswith()
to check if the string ends with a specific suffix.pythonfilename = "example.png" check = filename.endswith(".png") print(check)
This snippet checks if the string in
filename
ends with.png
. Since it does,endswith()
returnsTrue
.
Specifying Start and End of Search
endswith()
can also check within a specified range of characters by using the start
and end
parameters.
Choose a string to perform the check on.
Specify start and end indices.
Use
endswith()
with these indices to narrow down the check.pythontext = "Hello world" result = text.endswith("world", 6, 11) print(result)
By specifying indices 6 to 11, the method checks if "world" is at the end of the substring starting from index 6 up to but not including 11. It returns
True
as "world" indeed occupies that substring.
Checking Multiple Suffixes
endswith()
can accept a tuple of suffixes to check against, making it extremely versatile for multiple conditions.
Initialize a string variable.
Check if it ends with any of several suffixes by passing a tuple to
endswith()
.pythonfile_name = "photo.jpeg" is_valid = file_name.endswith((".png", ".jpg", ".jpeg")) print(is_valid)
This code checks whether
file_name
ends with any of the list of image file extensions. It printsTrue
because "photo.jpeg" ends with ".jpeg".
Practical Applications of endswith()
File Extension Validation
In file handling, ensuring that a file has the correct extension before processing can prevent many errors.
Retrieve or define file names.
Use
endswith()
to validate against expected file types.pythonfiles = ["report.txt", "image.png", "data.csv"] for file in files: if file.endswith((".txt", ".csv")): print(f"{file} is a valid document.") else: print(f"{file} is not a valid document.")
This loop goes through a list of file names, checking each against a tuple of valid document file extensions.
URL Processing
When dealing with web resources, sometimes you need to check URLs or paths for specific file types or markers.
Have a list or string of URLs to process.
Apply
endswith()
to detect if the URLs point to certain types of files.pythonurl = "https://example.com/image.png" if url.endswith(".png"): print("The URL points to a PNG image.") else: print("The URL does not point to a PNG image.")
Checks if the URL ends with ".png", indicating it's a PNG image, and processes accordingly.
Conclusion
The endswith()
method in Python is incredibly effective for string validation where the ending of the string is crucial, such as file formats, URL types, or specific terminologies in coding. By leveraging this method, enhance string processing capabilities to perform validations and checks more efficiently and reliably. While examples showcased involve file handling and URLs, the adaptability of endswith()
extends to any scenario where string suffixes play a pivotal role.
No comments yet.