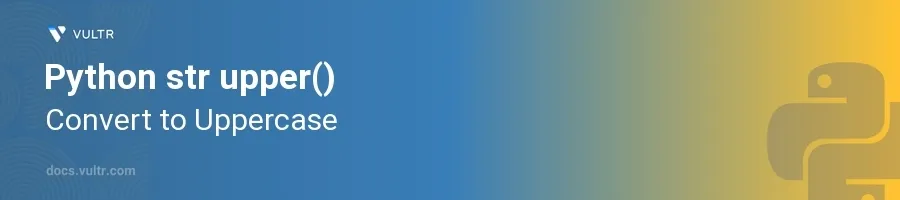
Introduction
The str.upper()
method in Python is an important string manipulation tool that converts all lowercase letters in a string to uppercase. This built-in method is straightforward yet powerful, making it ideal for formatting output, performing case-insensitive comparisons, and handling various data preprocessing tasks in text processing applications.
In this article, you will learn how to leverage the str.upper()
method in your Python scripts effectively. Explore various scenarios where this method proves to be vital, such as formatting user inputs, ensuring uniformity in data processing, and enhancing search functionalities in your applications.
Basic Usage of str.upper()
Convert String to Uppercase
Start with a simple string that contains both lowercase and uppercase characters.
Apply the
str.upper()
method.pythonoriginal_string = "Hello World" uppercase_string = original_string.upper() print(uppercase_string)
This code converts "Hello World" to "HELLO WORLD", demonstrating how
str.upper()
changes all lowercase letters in the string to uppercase.
Ensure Uniformity in User Inputs
Take user input that could vary in casing.
Convert the input to uppercase to standardize the data.
pythonuser_input = input("Enter your response: ") normalized_input = user_input.upper() print(normalized_input)
By converting user inputs to uppercase, maintain consistency regardless of how users enter their responses. This step is crucial for cases where user inputs are used in comparisons or stored in a database.
Advanced Applications of str.upper()
Case-Insensitive String Comparison
Address the issue of comparing strings that may differ in case.
Convert both strings to uppercase before performing the comparison.
pythonstring1 = "python" string2 = "Python" comparison_result = string1.upper() == string2.upper() print("Strings are equal:", comparison_result)
Here, both
string1
andstring2
are converted to uppercase, which facilitates a case-insensitive comparison, resulting in aTrue
output.
Enhancing Search Functionality
Implement a basic search functionality that overlooks case differences.
Convert both the text being searched and the search query to uppercase.
pythontext = "Searching through the document to find a specific word." search_query = "SPECIFIC" is_found = search_query.upper() in text.upper() print("Is the word found?", is_found)
This search checks for the presence of "SPECIFIC" in a given text, ignoring the original casing of words in the text and the search query.
Integrating str.upper() in Data Processing
Standardizing Data Entries
Handle diverse data entries that might include various cases.
Convert all string data to uppercase to facilitate uniform processing.
pythondata_entries = ["First Entry", "second entry", "THIRD ENTRY"] standardized_entries = [entry.upper() for entry in data_entries] print(standardized_entries)
This transformation ensures that all entries have uniform case, improving ease of processing and analysis, particularly when sorting or grouping data entries.
Preparing Data for Machine Learning Models
Prepare textual data for machine learning algorithms that require uniform input formats.
Convert text data to uppercase to reduce the variance due to letter cases.
pythonmachine_learning_input = "Natural Language Processing" processed_input = machine_learning_input.upper() print(processed_input)
This method ensures that the input for machine learning models is in a standard format, optimizing the model's ability to learn from the data.
Conclusion
The str.upper()
method in Python is a versatile tool for working with strings, especially when you need to standardize text. It is applicable in various fields such as data science, web development, and software engineering, where uniformity in text processing and case-insensitive comparisons are required. With the examples discussed above, harness the full potential of this method to improve the readability, consistency, and functionality of your Python programs.
No comments yet.