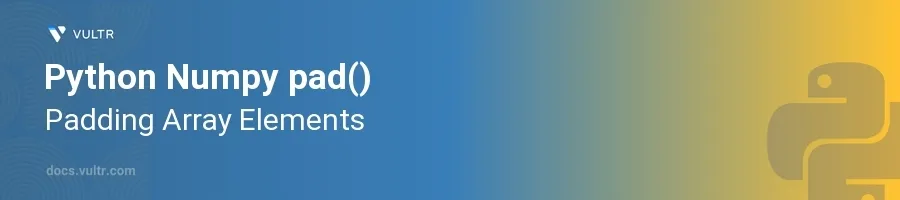
Introduction
The numpy.pad()
function in Python is a versatile tool used for padding arrays. Padding involves adding values around an existing array according to different modes and in varying widths, which can be crucial for various data preprocessing tasks in machine learning, image processing, and signal processing.
In this article, you will learn how to effectively use the pad()
function from the NumPy library to manipulate array dimensions. Explore how to apply padding to both one-dimensional and multi-dimensional arrays, understand different padding modes, and see practical examples showcasing the utility of array padding.
Understanding np.pad() Function
Purpose of Padding in Arrays
- Increase the size of array for alignment purposes.
- Prepare data that fits the input requirements of certain algorithms, particularly in machine learning and computer vision.
- Facilitate boundary condition management in computational applications.
Basic Syntax of np.pad()
Import the
numpy
library.Utilize the
np.pad()
function with its basic parameters.pythonimport numpy as np array = [1, 2, 3] padded_array = np.pad(array, pad_width=1, mode='constant', constant_values=0) print(padded_array)
Here, a one-dimensional array
array
is padded with0
on both sides. Thepad_width=1
specifies that one element should be added on each side. Themode='constant'
indicates that the padding should be a constant value specified byconstant_values=0
.
Using Different Padding Modes
Constant Padding
Understand that
constant
mode adds a constant value specified by the user.pythonarray = np.array([1, 2, 3]) constant_pad = np.pad(array, pad_width=2, mode='constant', constant_values=9) print(constant_pad)
This code adds two
9
s on both sides of the original array.
Edge Padding
Realize that
edge
mode pads with the edge values of the array.pythonedge_pad = np.pad(array, pad_width=1, mode='edge') print(edge_pad)
This approach replicates the edge values themselves, extending them outward.
Reflective Padding
Note that
reflect
mode mirrors the array around its edges.pythonreflect_pad = np.pad(array, pad_width=1, mode='reflect') print(reflect_pad)
This snippet creates a mirror effect by reflecting the array's values at its boundaries.
Wrap Padding
Understand that
wrap
mode wraps the array around.pythonwrap_pad = np.pad(array, pad_width=1, mode='wrap') print(wrap_pad)
In
wrap
mode, the array wraps around, letting the opposite end's values serve as padding.
Working with Multi-dimensional Arrays
Padding a Two-Dimensional Array
Extend the concept of padding to arrays with more than one dimension.
pythontwo_d_array = np.array([[1, 2], [3, 4]]) two_d_pad = np.pad(two_d_array, pad_width=1, mode='constant', constant_values=0) print(two_d_pad)
This resulting array shows zero-padding around the original 2D array, effectively creating a border similar to a frame.
Conclusion
The numpy.pad()
function is highly effective for manipulating the dimensions of arrays in Python. By understanding the detailed usage and different modes of padding, you can adjust arrays in a manner that suits a variety of applications, from image processing to complex data manipulations. Experiment with different padding modes and array configurations to optimize data preparation and algorithm performance in your projects, enhancing both versatility and code efficiency.
No comments yet.