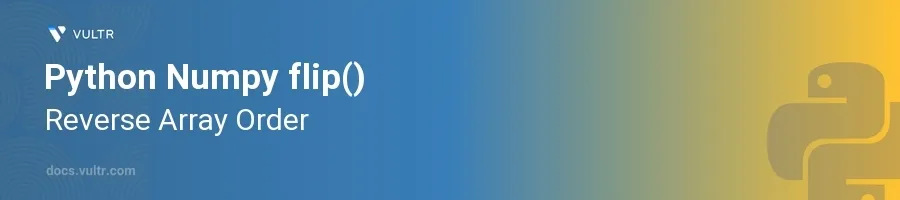
Introduction
The flip()
function in Python's NumPy library offers a straightforward and efficient way to reverse the order of array elements along a specified axis. This function is especially handy in data manipulation tasks where you need to rearrange data or develop algorithms that require an inverse perspective of the data.
In this article, you will learn how to apply the flip()
function to various types of arrays. Explore the functionality of reversing both one-dimensional and multi-dimensional arrays and understand the impact of specifying different axes for multi-dimensional data.
Reversing One-Dimensional Arrays
Basic Reversal of 1D Array
Import the NumPy library.
Create a one-dimensional array.
Use the
flip()
function to reverse the array.pythonimport numpy as np arr_1d = np.array([1, 2, 3, 4, 5]) reversed_arr_1d = np.flip(arr_1d) print(reversed_arr_1d)
This code reverses the order of elements in
arr_1d
. Theflip()
function treats the entire array as its input and flips the order of its elements.
Reversing Multi-Dimensional Arrays
Reversing Along Specific Axes
Create a two-dimensional array.
Reverse the array along different axes using
flip()
.Analyze the output to understand axis-specific flipping.
pythonarr_2d = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]]) reversed_arr_2d_axis0 = np.flip(arr_2d, axis=0) reversed_arr_2d_axis1 = np.flip(arr_2d, axis=1) print("Reversed along axis 0:\n", reversed_arr_2d_axis0) print("Reversed along axis 1:\n", reversed_arr_2d_axis1)
In this example:
reversed_arr_2d_axis0
flips the rows of the array (i.e., the first row becomes the last).reversed_arr_2d_axis1
flips the columns of the array (i.e., the first column becomes the last).
Handling Complex Reversal Patterns
Simultaneous Reversal on Multiple Axes
Utilize NumPy to create a three-dimensional array.
Apply
flip()
to reverse the array along more than one axis.pythonarr_3d = np.array([[[1, 2], [3, 4]], [[5, 6], [7, 8]]]) reversed_arr_3d = np.flip(arr_3d, axis=(0, 2)) print("Original array:\n", arr_3d) print("Reversed array along axes 0 and 2:\n", reversed_arr_3d)
This snippet reverses the elements along the first and the last axes of a 3D array, showing the flexibility of
flip()
in handling complex multi-dimensional data.
Conclusion
The flip()
function in NumPy is a powerful tool for reversing the order of numpy array elements, providing flexibility across different shapes and dimensions of data. It simplifies tasks requiring data manipulation and inversion of order which can be crucial for certain data processing applications. By mastering flip()
, you equip yourself with a versatile function capable of handling various data reversal scenarios, ultimately enhancing data manipulation skills and capabilities in Python. Use these examples as a guide to implement array reversals effectively in your projects.
No comments yet.