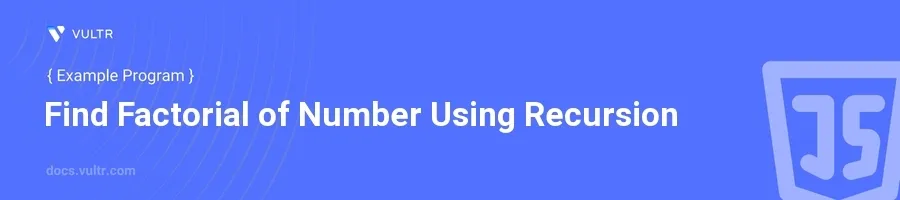
Introduction
Calculating the factorial of a number is a common mathematical task, frequently required in various programming scenarios. A factorial of a positive integer n, denoted by n!, is the product of all positive integers less than or equal to n. This concept finds extensive use in areas like combinatorics, algebra, and mathematical analysis.
In this article, you will learn how to implement a factorial function using recursion in JavaScript. Follow through with detailed examples to understand the recursive approach and how it executes to compute factorial values effectively.
Understanding Factorial Calculation Through Recursion
Basic Concept of Recursion
- Recognize that recursion is a method where the function calls itself to solve its instance.
- Understand that a recursive function needs a base case to stop the recursion, preventing it from executing indefinitely.
- Learn that in the case of factorial calculation, the recursive relation is n! = n * (n-1)!, and the base case is typically 0! = 1.
Writing the Recursive Function
Define a JavaScript function named
factorial
that accepts one parametern
, the number whose factorial is required.Within the function, establish the base case. If
n
is 0, return 1.Implement the recursive step: return
n
multiplied by the factorial ofn - 1
.javascriptfunction factorial(n) { if (n === 0) { return 1; } return n * factorial(n - 1); }
In this code, the function checks if
n
is 0 (the base case), and returns 1. If not, it calculates the factorial by calling itself withn - 1
until it reaches the base case.
Testing the Function with Various Inputs
It's important to test the function with several different values.
Start with
factorial(0)
, which should return1
as per the definition of 0!.javascriptconsole.log("Factorial of 0:", factorial(0)); // Output will be 1
Test with
factorial(5)
, expecting a result of 120 because 5! = 5 * 4 * 3 * 2 * 1 = 120.javascriptconsole.log("Factorial of 5:", factorial(5)); // Output should be 120
Try a higher number like
10
and validate the result.javascriptconsole.log("Factorial of 10:", factorial(10)); // Output should be 3628800
Conclusion
The factorial of a number calculated via recursion in JavaScript provides a clear and effective way of understanding both the factorial operation and recursive programming. By breaking the problem into smaller, manageable tasks (each factorial calling for another factorial calculation), recursion offers a structured solution. The examples highlighted ensure you have a robust concept of implementing and validating this recursive method. Consider using this recursive approach to compute factorials or similar recursive calculations effectively in your JavaScript projects.
No comments yet.