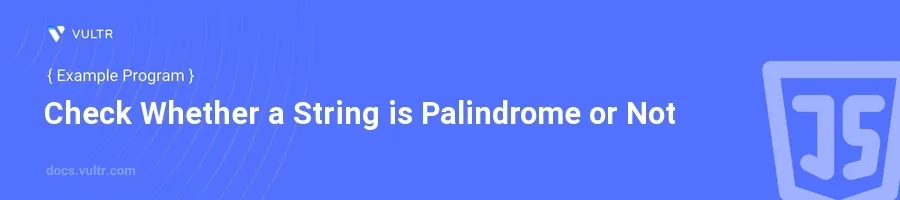
Introduction
A palindrome is a word, phrase, number, or other sequences of characters that reads the same forward and backward, ignoring spaces, punctuation, and capitalization. Checking if a string is a palindrome is a popular problem in computer science and programming interviews.
In this article, you will learn how to check whether a string is a palindrome using JavaScript. You'll explore multiple examples that demonstrate how to implement this function and discuss improvements to handle edge cases effectively.
Basic Palindrome Check
Implementing a Simple Palindrome Function
Create a function to reverse the input string.
Compare the original string with the reversed string to determine if it's a palindrome.
javascriptfunction isPalindrome(str) { const reversed = str.split('').reverse().join(''); return str === reversed; }
This function splits the string into an array of characters, reverses that array, and joins the characters back into a string. It then compares this string to the original string.
Examples of Basic Palindrome Check
Test the function with a palindrome.
Test the function with a non-palindrome.
javascriptconsole.log(isPalindrome('racecar')); // Output: true console.log(isPalindrome('hello')); // Output: false
Here, 'racecar' reads the same backward and forward, whereas 'hello' does not.
Advanced Palindrome Check
Handling Case Sensitivity and Non-Alphanumeric Characters
Modify the original function to convert the string to lowercase.
Use a regular expression to remove non-alphanumeric characters.
javascriptfunction isAdvancedPalindrome(str) { const cleaned = str.toLowerCase().replace(/[\W_]/g, ''); const reversed = cleaned.split('').reverse().join(''); return cleaned === reversed; }
This version of the function first converts the entire string to lowercase and removes any characters that aren't letters or numbers. It then checks if the cleaned, reversed string is a palindrome.
Examples of Advanced Palindrome Check
Test the function with a complex string including spaces and punctuation.
Test the function with mixed cases.
javascriptconsole.log(isAdvancedPalindrome('A man, a plan, a canal: Panama')); // Output: true console.log(isAdvancedPalindrome('No lemon, no melon')); // Output: true
These strings, when spaces and punctuation are removed and the case is normalized, read the same backward as forward.
Conclusion
By following the examples above, you can effectively determine if a string is a palindrome in JavaScript. Starting from a basic implementation to more advanced checks that handle cases and non-alphanumeric characters, these techniques ensure that your function is robust and handles a wider range of inputs. Use these methods in your projects to efficiently handle string evaluation tasks relating to palindromes.
No comments yet.