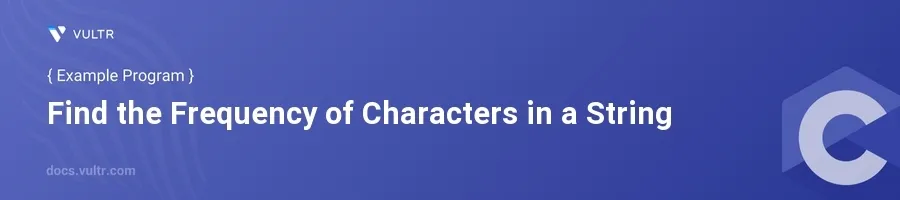
Introduction
The process of counting the frequency of characters in a string is a fundamental programming task that often appears in software development, data analysis, and algorithm design. This entails scanning a string and counting how many times each character appears in it, which can be crucial for tasks such as data validation, cryptography, and text analysis.
In this article, you will learn how to implement a program in C that determines the frequency of each character in a given string. Delve into practical examples that show how to effectively count character occurrences using arrays and basic loops in C.
Setting Up the Frequency Counter
Initialize the Frequency Array
Define a character array for the input string.
Initialize an integer array to store the frequency of each character.
cchar str[100]; int freq[256] = {0}; // 256 for all ASCII characters
This code sets up a string
str
where the input will be stored and an arrayfreq
initialized to zero to count the occurrences of each ASCII character.
Get User Input
Prompt the user to enter a string.
Store the input in the character array.
cprintf("Enter a string: "); fgets(str, sizeof(str), stdin);
The
fgets
function reads a line fromstdin
and stores it into the stringstr
, ensuring that the input does not exceed the buffer size.
Counting Characters in the String
Loop Through Each Character
Use a loop to traverse each character in the string until the null character is encountered.
Increase the corresponding index in the frequency array.
cint i = 0; while (str[i] != '\0') { if (str[i] != '\n') { // To ignore newline character from fgets freq[(int)str[i]]++; } i++; }
This loop increments the count for each character's ASCII value in the
freq
array. It ignores the newline character that might be read byfgets
.
Display the Frequencies
Output the Non-zero Frequencies
Loop through the frequency array.
Print only the characters that appear at least once.
cfor (int i = 0; i < 256; i++) { if (freq[i] != 0) { printf("'%c' occurs %d times in the string.\n", i, freq[i]); } }
This code iterates over each possible character value, and when a non-zero frequency is found, it prints the character and its count.
Conclusion
By implementing this program in C, you harness basic array usage and looping constructs to solve the practical problem of counting character occurrences. This approach efficiently tracks the frequencies of characters in a string using their ASCII values as direct indices in an array, allowing for a concise and fast execution. Use these techniques to further explore text processing tasks or as a stepping stone to more complex data counting and manipulation methods in your future projects.
No comments yet.