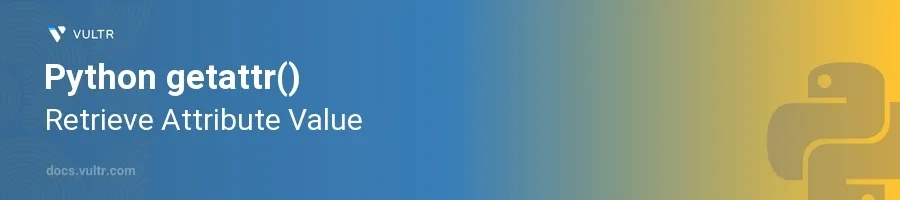
Introduction
The getattr()
function in Python provides a dynamic way of accessing the attributes of an object. This built-in function retrieves the value of a named attribute from an object, allowing for flexible code implementations where attributes might not be known during the code-writing phase. It’s especially useful in scenarios where object attributes are managed dynamically or when interfacing with external systems.
In this article, you will learn how to effectively use the getattr()
function to access attributes dynamically. Explore various examples to understand how to handle default values and avoid common errors when attributes are not present in the object.
Retrieving Simple Attributes
Access an Existing Attribute
Create an object or use an existing one.
Specify the attribute name as a string.
Use
getattr()
to retrieve the value.pythonclass Person: def __init__(self, name, age): self.name = name self.age = age person = Person("Alice", 30) name = getattr(person, 'name') print(name)
This code successfully retrieves the name of the person object, which is "Alice".
Handle Non-existent Attributes
Understand the risk of
AttributeError
if the attribute does not exist.Use the optional third argument of
getattr()
to specify a default value.Provide the default value to avoid an exception.
pythonage = getattr(person, 'height', 'No height specified') print(age)
Here, since the 'height' attribute does not exist,
getattr()
returns the default value "No height specified".
Working with Methods
Call a Method Using getattr()
Realize that methods are also attributes of an object that can be retrieved.
Use
getattr()
to obtain a method reference.Call the method after retrieving it.
pythonget_name = getattr(person, 'name') print(get_name())
In this example,
getattr()
is used to retrieve thename
method of theperson
object. After the retrieval, the method is called to print "Alice".
Use in Advanced Scenarios
Dynamic Attribute Handling
Consider a situation where attribute names are coming as input from users or external systems.
Use
getattr()
dynamically to fetch these attributes.Implement error handling or default values to avoid exceptions.
pythonattributes = ['name', 'age', 'job'] for attribute in attributes: value = getattr(person, attribute, f'No {attribute} specified') print(f'{attribute}: {value}')
This loop dynamically retrieves the value of each attribute listed in the
attributes
list. If an attribute is not present in the person object, it prints a customized message indicating so.
Conclusion
The getattr()
function in Python is a powerful tool for dynamic attribute access in objects. It enables you to retrieve the value of attributes whose names might not be known until runtime, enhancing the flexibility of your code. By utilizing getattr()
with the proper handling of default values and potential errors, you can write more robust and adaptable Python applications. Explore this function further to enrich your projects with dynamic capabilities.
No comments yet.