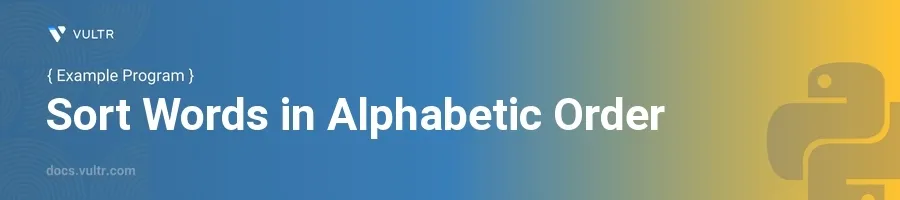
Introduction
Sorting words alphabetically is a common task in programming, particularly important when organizing data, enabling better search efficiency, and improving readability. This operation involves rearranging a list of words so that they appear in a sequence from A to Z, similar to how words are listed in a dictionary.
In this article, you will learn how to sort words in alphabetic order using Python. You will explore different methods and scenarios, including sorting words with case sensitivity and case insensitivity, and see examples employing both the built-in sort()
method and the sorted()
function of Python.
Basic Sorting of Words
Sort Using the sort() Method
Create a list of words.
Use the
sort()
method to alphabetically sort the list.pythonwords = ["banana", "apple", "cherry"] words.sort() print(words)
Here,
words.sort()
modifies the original listwords
by sorting the elements in alphabetical order. When printed, the list displays['apple', 'banana', 'cherry']
.
Sort Using the sorted() Function
Create a list of words.
Apply the
sorted()
function and store the result.pythonwords = ["banana", "apple", "cherry"] sorted_words = sorted(words) print(sorted_words)
The
sorted()
function returns a new list that is a sorted version of the input list, thus the original listwords
remains unchanged. The output shows the words sorted alphabetically.
Handling Case Sensitivity
Case Insensitive Sorting
Create a list containing words with mixed case letters.
Use the
sorted()
function with the key argument set tostr.lower
.pythonwords = ["Banana", "apple", "Cherry"] sorted_words = sorted(words, key=str.lower) print(sorted_words)
This approach uses
str.lower
as the key function, which converts all characters to lowercase before performing the sort, leading to a case-insensitive alphabetical order in the output.
Advanced Sorting Techniques
Sorting Words by Length
Understand that sorting can be customized beyond simple alphabetical order.
Create a list of words.
Use
sorted()
with thekey
parameter to sort words according to their length.pythonwords = ["banana", "apple", "cherry", "date"] sorted_words = sorted(words, key=len) print(sorted_words)
This snippet sorts the words based on their length. The
key=len
ensures that the sorting criterion is the length of the words, not the alphabetical order.
Combining Alphabetical and Length-based Sorting
When sorting by multiple criteria, use a tuple in the
key
function.Sort primarily by length, then by alphabetical order for words of the same length.
pythonwords = ["banana", "apple", "cherry", "fig", "egg"] sorted_words = sorted(words, key=lambda word: (len(word), word)) print(sorted_words)
The lambda function here returns a tuple, with the first element being the length of each word and the second the word itself. Python sorts primarily by the first tuple element, and if there are ties, it sorts by the second element, ensuring a sequential order both by length and alphabet.
Conclusion
Sorting words in Python can be achieved through various methods, tailored to specific requirements like case sensitivity or additional sorting criteria like word length. Utilize the sort()
method for in-place sorting when you need to alter the original list, or the sorted()
function when a new sorted list is required. With the strategies discussed, you can efficiently organize textual data to suit your program's needs, enhancing functionality and user experience.
No comments yet.