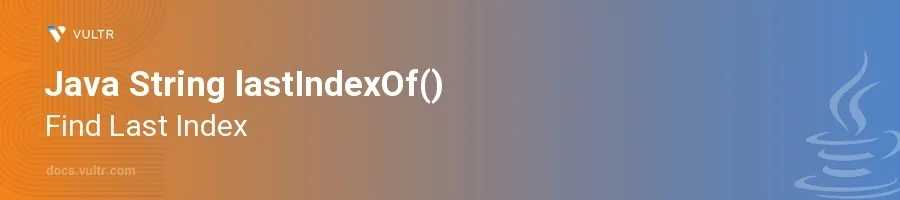
Introduction
The lastIndexOf()
method in Java is a part of the String
class and is used to find the position of the last occurrence of a specified character or substring within a string. This method proves vital in text processing where you need to navigate or manipulate strings based on certain characters or phrases from the end towards the beginning. It facilitates tasks like parsing file paths, handling extensions, or searching for specific data patterns in user inputs or files.
In this article, you will learn how to use the lastIndexOf()
method in Java. This includes finding the last index of characters and substrings, understanding its overloads, and leveraging it in practical programming scenarios. By mastering lastIndexOf()
, you enhance your ability to efficiently handle string manipulations in Java applications.
Using lastIndexOf() to Find Character Positions
Find the Last Index of a Character
Start with a string where you want to find a character's last occurrence.
Utilize the
lastIndexOf(int ch)
method to get the position.javaString exampleStr = "hello world"; int lastIndex = exampleStr.lastIndexOf('o'); System.out.println("Last index of 'o': " + lastIndex);
This code snippet finds the last occurrence of the character 'o' in the string "hello world". The
lastIndexOf()
method returns7
, which is the zero-based index position of the last 'o'.
Find Last Index with Character Unicode
Focus on a scenario where you need the Unicode form of the character.
Apply the
lastIndexOf(int ch)
method using the Unicode value of the character.javaString unicodeStr = "funny bunny"; int lastIndexUnicode = unicodeStr.lastIndexOf(121); // Unicode for 'y' System.out.println("Last index of 'y' using Unicode: " + lastIndexUnicode);
Here, the Unicode value
121
corresponds to 'y'. The method locates the last 'y' in the string "funny bunny" at index10
.
Using lastIndexOf() with Substrings
Finding the Last Index of a Substring
Create a string where a specific substring’s last occurrence needs identification.
Use the
lastIndexOf(String str)
method.javaString phrase = "The quick brown fox jumps over the lazy dog"; int substrIndex = phrase.lastIndexOf("the"); System.out.println("Last index of substring 'the': " + substrIndex);
In this instance,
lastIndexOf()
finds the last occurrence of the substring "the", returning31
. This method is case-sensitive which is why it didn't match the "The" at the beginning of the string.
Handling Case Insensitivity
Adjust your approach when case sensitivity is an issue.
Convert the entire string and the search substring to the same case (either lower or upper) before searching.
javaString casePhrase = "Enjoy The Silence"; int caseInsensitiveIndex = casePhrase.toLowerCase().lastIndexOf("the"); System.out.println("Case insensitive last index of 'the': " + caseInsensitiveIndex);
This technique ensures that the search ignores differences in case, thereby making the method effectively case-insensitive. The
toLowerCase()
converts all characters of the string into lower case, thenlastIndexOf()
is used to find "the".
Advanced Usage with Offset
Searching for a Substring Starting from a Given Index
Sometimes, the search needs to be limited to a specific part of the string.
Use the
lastIndexOf(String str, int fromIndex)
method, which starts the search backward from the specified position.javaString detailedPhrase = "She sells sea shells on the sea shore"; int offsetIndex = detailedPhrase.lastIndexOf("sea", 25); System.out.println("Last index of 'sea' starting from index 25: " + offsetIndex);
The example limits the backward search for "sea" up to the 25th position of the string. This method finds the first occurrence of "sea" located before the mentioned index from the end, which is at position
18
.
Conclusion
By exploring the lastIndexOf()
method in Java's String
class, you gain the ability to effectively search strings for the last occurrences of characters or substrings. Whether working with simple characters, addressing case sensitivity, or using advanced searching with offsets, this method offers robust solutions for handling string manipulations. Harness these different usages of lastIndexOf()
to optimize your Java applications, ensuring efficient and powerful text processing capabilities tailored to fit various programming needs.
No comments yet.