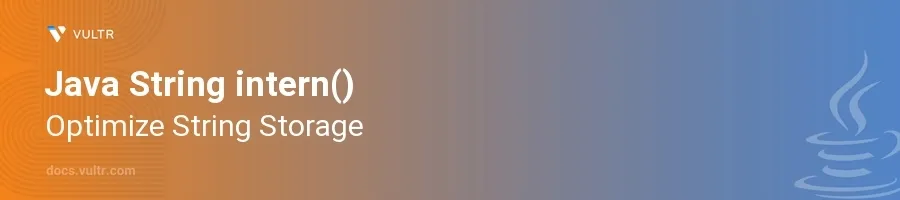
Introduction
The intern()
method in Java’s String class is pivotal for optimizing memory usage and string management within Java applications. This method helps maintain a single copy of distinct string literals in the string pool, which is part of the Java heap memory. Leveraging intern()
can dramatically reduce memory consumption, especially in applications where many identical strings are used repeatedly.
In this article, you will learn how to utilize the intern()
method effectively in various Java programming scenarios. You'll explore how this method can be applied to optimize string storage, reduce memory overhead, and improve application performance through practical examples and explanations.
Understanding String Interning
Basics of String Interning
- Recognize that the JVM maintains a special memory region known as the "intern pool."
- Learn that strings that are explicitly interned or derived from literals are stored in this pool.
How intern() Works
- Understand that calling
intern()
on a String instance checks if an equal string already exists in the pool. - Know that if it exists, it returns the reference from the pool; otherwise, it adds the string to the pool and returns its reference.
Advantages of Using intern()
- Reduced memory consumption as duplicate string literals share a single storage space.
- Increased performance in string comparison using
==
instead ofequals()
, because interned strings share the same reference.
Using intern() in Java Code
Interning New String Objects
Create a new string instance using the
new
keyword.Use the
intern()
method to add or retrieve the string from the pool.javaString s1 = new String("Example"); String s2 = s1.intern();
Starting with
s1
, a new string is created, ands2
will reference the interned version of the string from the intern pool. If "Example" was already in the intern pool,s2
will reference the pre-existing pooled instance.
Demonstrating Memory Efficiency
Instantiate multiple strings explicitly and intern them.
Compare the memory address of interned strings to showcase their efficiency.
javaString str1 = new String("Hello").intern(); String str2 = new String("Hello").intern(); System.out.println(str1 == str2); // This will print true
Here,
str1
andstr2
are both directed to the same reference in the memory thanks to the intern pool. The==
comparison returns true, indicating they are indeed the same object, which wouldn't be the case without interning.
Performance Impact
Use interned strings in a context where performance is critical.
Observe the improvement in performance notably in scenarios involving extensive string manipulation and comparison.
javaString constantStr = "World"; String newString = new String("World").intern(); long startTime = System.nanoTime(); for(int i = 0; i < 1000000; i++) { if(constantStr == newString) { continue; } } long endTime = System.nanoTime(); System.out.println("Duration: " + (endTime - startTime) + " nanoseconds");
This code measures the time taken to perform 1,000,000 quick reference checks between two interned strings.
Best Practices and Considerations
When to Use intern()
- Use
intern()
when an application heavily relies on string comparisons or requires optimized memory usage for a large number of equal strings. - Apply string interning mostly for strings that are sure to have duplicates.
Possible Pitfalls
- Excessive use of
intern()
can lead to filling up the PermGen (or Metaspace in Java 8 and above), which stores the interned strings, leading to performance degradation. - Evaluate memory versus performance trade-offs before deciding to intern strings, especially in environments with limited resources.
Conclusion
Using the intern()
method intelligently in Java can help optimize both memory usage and performance. It ensures minimal string objects are maintained within the JVM by pooling common strings. While intern()
is powerful, understand when and where to use it to prevent potential issues like memory overflow in the intern pool. Implement the string interning concepts and examples provided here to manage strings more efficiently and to boost your Java application's performance.
No comments yet.