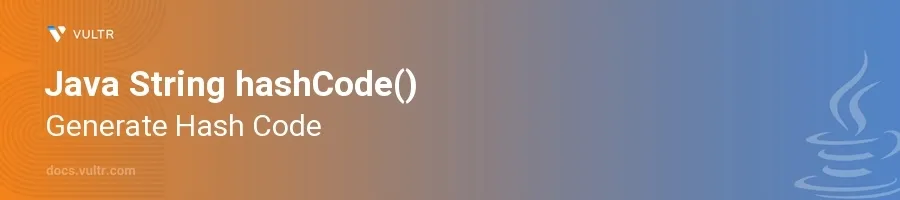
Introduction
The hashCode()
method in Java's String class is a crucial tool for generating integer hash codes. Essentially, this method is used widely in hashing data structures like hash tables or hash maps, where it assists in determining the bucket location for storing String objects efficiently. Understanding how to properly utilize this function is key in optimizing data retrieval processes and ensuring a balanced load across data structures.
In this article, you will learn how to harness the power of the hashCode()
method in various practical contexts. Explore strategies for implementing and validating hash codes, and discover how these can influence the performance and reliability of Java applications.
Understanding hashCode() in Java
The Basics of String Hash Code
Recognize that the
hashCode()
method for a string is computed using the string contents.Use the hashCode method to retrieve the hash code of a string.
javaString example = "SampleString"; int hashValue = example.hashCode(); System.out.println("Hash Code: " + hashValue);
Here,
hashCode()
calculates the hash code based on the content ofexample
. Each character's code is used in the calculation, ensuring that the same string always produces the same hash code within the same session or across different Java sessions.
Significance of Consistent Hash Codes
Note that consistent hash codes are vital for the performance of hash-based collections.
Understand that if
hashCode()
is overridden,equals()
should also be overridden to maintain the general contract for theObject
class.javaString str1 = "Hello"; String str2 = new String("Hello"); System.out.println(str1.hashCode() == str2.hashCode()); System.out.println(str1.equals(str2));
In this code, despite
str2
being a new instance, its hash code matches that ofstr1
because their contents are identical. The output of both print statements will betrue
, demonstrating the consistency of hash codes across different string instances with the same contents.
Collisions and Their Impact
Acknowledge that hash code collisions (where different strings have the same hash code) can occur but are relatively rare for the
hashCode()
method.Learn about how Java handles collisions internally using linked lists or tree structures in hash tables.
There isn't a specific code snippet provided here but know that, internally, collections like
HashMap
andHashSet
manage collisions efficiently to maintain optimal performance even in the case of hash code collisions.
Advanced Uses of String Hash Codes
Customizing Hash Functions for Specific Needs
Consider the possibility of implementing a custom hash function if the distribution of
hashCode()
outputs isn't optimal for specific cases.Ensure that the custom hash function adheres to the contract that equal objects must produce the same hash code.
javaclass CustomString { private String content; CustomString(String content) { this.content = content; } @Override public int hashCode() { // Simple custom hash function (just an example, not recommended for production) return this.content.length(); } }
In this custom class
CustomString
, the hash code is simplified to return the length of the string. This is merely illustrative and should be adjusted for actual use-cases to avoid high collision rates.
Debugging Hash Code Issues
If unexpected behavior in a collection occurs, verify that
hashCode()
returns consistent results.Debug by logging or outputting hash codes in environments where consistency seems to fluctuate, identifying any deviations in expected behavior.
Outputting the hash codes during runtime can identify where inconsistencies begin, especially useful when dealing with complex systems where the string contents might be changing unexpectedly.
Conclusion
Java's hashCode()
method is an essential component of the String class, playing a critical role in the performance and efficiency of hash-based collections. By exploring how to effectively apply this method, as well as understanding its underlying mechanics and potential pitfalls, you enhance the stability and speed of Java applications. Employ the strategies discussed to ensure robust implementations of hash codes, allowing for optimized data management and retrieval in your Java projects.
No comments yet.