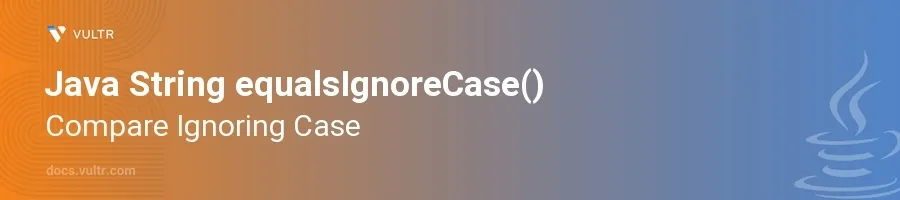
Introduction
Java provides several methods for comparing strings to enhance flexibility and efficiency in handling text data. Among these, the equalsIgnoreCase()
method stands out when you need to compare two strings without considering their case sensitivity. This capability is particularly useful in user interfaces and data processing where the case of characters shouldn't influence the logic or outcomes of the operations.
In this article, you will learn how to effectively leverage the equalsIgnoreCase()
method in Java. Explore various examples where this method becomes invaluable, understand its behavior with different inputs, and compare its use with other string comparison techniques.
Understanding equalsIgnoreCase()
Basic Usage of equalsIgnoreCase()
Start by creating two strings where one contains upper case letters and the other lower case.
Use the
equalsIgnoreCase()
method to compare these two strings.javaString str1 = "java"; String str2 = "JAVA"; boolean result = str1.equalsIgnoreCase(str2); System.out.println(result);
This code demonstrates a basic case-insensitive comparison. Despite
str1
being in lower case andstr2
in upper case,equalsIgnoreCase()
evaluates them as equal and returnstrue
.
Comparing equalsIgnoreCase() with equals()
Understand that the
equals()
method is case-sensitive whileequalsIgnoreCase()
is not.Prepare two strings, identical in content but different in case.
Compare them using both
equals()
andequalsIgnoreCase()
.javaString str3 = "Hello"; String str4 = "hello"; System.out.println("Using equals: " + str3.equals(str4)); System.out.println("Using equalsIgnoreCase: " + str3.equalsIgnoreCase(str4));
Here, the output of
equals()
will befalse
due to case difference, whileequalsIgnoreCase()
will outputtrue
, showing its utility in case-insensitive comparisons.
Scenario Example: User Input Validation
Consider a situation where user input for a command or choice should not be case-sensitive.
Simulate such a scenario to validate user input using
equalsIgnoreCase()
.javaString command = "Start"; String userInput = "start"; if (command.equalsIgnoreCase(userInput)) { System.out.println("Command recognized. Starting process..."); } else { System.out.println("Invalid command."); }
In this example, even though the user types "start" in lower case, the program recognizes it as valid due to the
equalsIgnoreCase()
method. This is particularly useful in making user interfaces friendlier and less prone to user error.
Advanced Considerations
Performance Implications
- Discuss that
equalsIgnoreCase()
may involve extra processing to handle case conversion. - Note that for very large strings or highly performance-sensitive applications, this should be considered.
Despite these considerations, equalsIgnoreCase()
is generally efficient for typical use-cases involving String comparisons in Java.
Null-Handling
Remember that invoking
equalsIgnoreCase()
on a null String will result in aNullPointerException
.Always ensure the reference variables you compare are not null before using this method.
javaString str5 = null; String str6 = "test"; // Uncomment the following line to see the NullPointerException // System.out.println(str5.equalsIgnoreCase(str6));
Avoid this error by adding null checks before invoking comparison methods on strings possibly holding null.
Conclusion
The equalsIgnoreCase()
function in Java is an essential tool for string comparison that disregards the case of characters. Its ease of use and ability to prevent common user errors make it an indispensable method in Java programming. Utilizing equalsIgnoreCase()
effectively ensures that applications remain robust and user-friendly, especially in scenarios involving textual data and user interfaces. By embracing the techniques explored, you enhance your Java programs to handle string comparisons more inclusively and efficiently.
No comments yet.