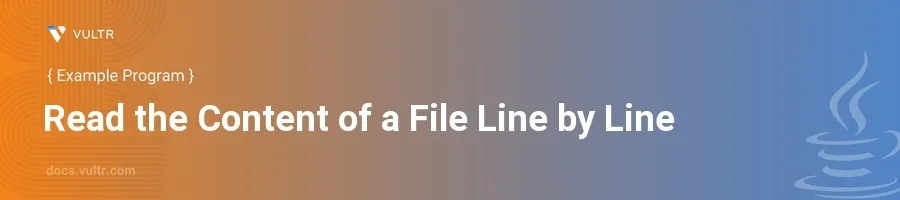
Introduction
Reading a file line by line is a common operation in Java programming, especially when dealing with text processing, logfile analysis, or configuration file parsing. Java provides numerous ways to perform this task, each suitable for different scenarios depending on the file size, type of data, or specific requirements like encoding types.
In this article, you will learn how to read content from a file line by line using various methods in Java. You will explore the traditional BufferedReader
class, the modern java.nio
package, and even look into Java 8's Streams API. Each method provides a practical example to ensure you grasp how to implement it in real-world projects.
Using BufferedReader
Basic File Reading
Import the necessary classes from the Java I/O library.
Open a file using
FileReader
wrapped byBufferedReader
.Read the file line by line in a loop until all lines are processed.
javaimport java.io.BufferedReader; import java.io.FileReader; import java.io.IOException; public class FileReaderExample { public static void main(String[] args) { String path = "example.txt"; try (BufferedReader reader = new BufferedReader(new FileReader(path))) { String line; while ((line = reader.readLine()) != null) { System.out.println(line); } } catch (IOException e) { e.printStackTrace(); } } }
In this example,
BufferedReader
reads the fileexample.txt
line by line.readLine()
returns null when end-of-file (EOF) is reached, terminating the loop. This method is efficient and simple for reading text files line by line.
Exception Handling
Use the try-with-resources statement to handle resource management and exceptions automatically.
The example above demonstrates the use of try-with-resources, which ensures that the
BufferedReader
instance closes properly even if an exception occurs. This method handlesIOException
, which must be managed properly to avoid resource leaks and other bugs.
Using java.nio.file Files Class
Reading Using Stream API
Utilize Java 8's Stream API to efficiently process large files.
Utilize
Files.lines(Path)
to get a stream of lines directly from the file.javaimport java.nio.file.Files; import java.nio.file.Paths; import java.util.stream.Stream; public class NioFileReadExample { public static void main(String[] args) { String filePath = "example.txt"; try (Stream<String> lines = Files.lines(Paths.get(filePath))) { lines.forEach(System.out::println); } catch (IOException e) { e.printStackTrace(); } } }
This code sample demonstrates reading a file line by line using the
Files.lines
method in Java NIO. This approach returns aStream<String>
making it possible to use the powerful stream operations for processing the file content. It’s especially useful for large files or when performing complex filtering and manipulation of file data.
Using Scanner Class
Read with Delimiters
Deploy
Scanner
for its flexibility with delimiters and its ability to parse primitives directly from text input.Configure
Scanner
to use a newline delimiter for reading the file line by line.javaimport java.io.File; import java.io.FileNotFoundException; import java.util.Scanner; public class ScannerExample { public static void main(String[] args) { File file = new File("example.txt"); try (Scanner scanner = new Scanner(file)) { scanner.useDelimiter(System.lineSeparator()); while (scanner.hasNext()) { System.out.println(scanner.next()); } } catch (FileNotFoundException e) { e.printStackTrace(); } } }
This example uses
Scanner
, a flexible reader that can be configured to use different delimiters, making it suitable for reading files that may not strictly be line-based or require special parsing. In this scenario, it uses the system-dependent line separator as the delimiter.
Conclusion
You have now explored several methods to read files line by line in Java. Each method has its own advantages. For example, BufferedReader
offers straightforward line-by-line reading, Files.lines
supports functional-style operations, while Scanner
provides parsing capabilities. Choose the approach that best fits the needs of your application based on the file size, type, and specific processing requirements. Implementing these methods correctly ensures efficient and robust file reading capabilities in your Java programs.
No comments yet.