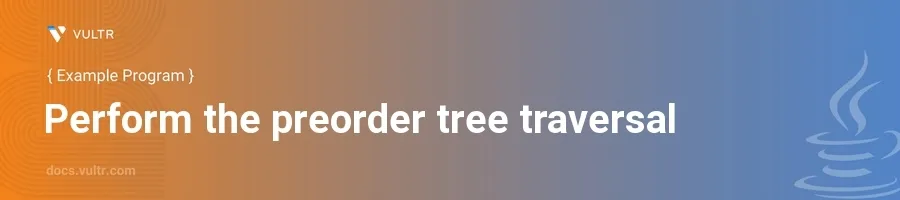
Introduction
Preorder tree traversal is a type of depth-first traversal method used in trees, where the current node is processed before its child nodes. It's a fundamental technique in computer science used in various applications including expression parsing, syntax tree traversal, and in the strategies for coping with hierarchical data structures.
In this article, you will learn how to perform preorder tree traversal in Java. Explore practical examples to understand how to implement this traversal technique in both recursive and iterative approaches. Each section is accompanied by sample code snippets, ensuring you grasp the procedure efficiently.
Recursive Preorder Traversal
In the recursive approach to preorder traversal, the process involves visiting the node first, then recursively traversing the left subtree, and finally, the right subtree. This is the most straightforward method to implement and understand.
Implementing Recursive Preorder Traversal
Define a TreeNode class for structure.
Create the recursive function to handle the traversal.
javaclass TreeNode { int value; TreeNode left, right; TreeNode(int value) { this.value = value; left = right = null; } } public class BinaryTree { TreeNode root; void preorderTraversal(TreeNode node) { if (node == null) { return; } System.out.print(node.value + " "); preorderTraversal(node.left); preorderTraversal(node.right); } }
In this Java class,
TreeNode
defines the node structure with integer values. ThepreorderTraversal()
method applies the recursion. First, it prints the current node's value, then it calls itself to traverse the left and then right child nodes.
Execution in a Main Method
Construct a simple binary tree.
Call the
preorderTraversal
method to print its nodes.javapublic static void main(String[] args) { BinaryTree tree = new BinaryTree(); tree.root = new TreeNode(1); tree.root.left = new TreeNode(2); tree.root.right = new TreeNode(3); tree.root.left.left = new TreeNode(4); tree.root.left.right = new TreeNode(5); tree.preorderTraversal(tree.root); }
This
main
method sets up a basic tree and prints out the nodes in preorder. The output for this particular tree configuration will be1 2 4 5 3
.
Iterative Preorder Traversal
Though recursion is elegant, it can be less efficient and riskier (stack overflow) for deep trees. The iterative approach utilizes a stack to mimic the recursion process without the actual recursive calls.
Implementing Iterative Preorder Traversal
Use a Stack data structure.
Implement the traversal by manually managing the node order.
javaimport java.util.Stack; public class BinaryTree { void iterativePreorder(TreeNode node) { if (node == null) { return; } Stack<TreeNode> stack = new Stack<>(); stack.push(node); while (!stack.empty()) { TreeNode currentNode = stack.pop(); System.out.print(currentNode.value + " "); if (currentNode.right != null) { stack.push(currentNode.right); } if (currentNode.left != null) { stack.push(currentNode.left); } } } }
In this approach, nodes are manually pushed to and popped from a stack to control the order of node processing. The right child is pushed first to ensure that the left child is processed first, adhering to the preorder rules.
Conclusion
Performing preorder tree traversal in Java can be approached in various ways, each offering unique advantages. The recursive method is simpler and more intuitive, while the iterative method provides more control and efficiency, especially useful in large, deep trees. By mastering these traversal techniques, you enhance your ability to work with and manipulate complex data structures in Java, which is critical for solving advanced problems in software development and systems design.
No comments yet.