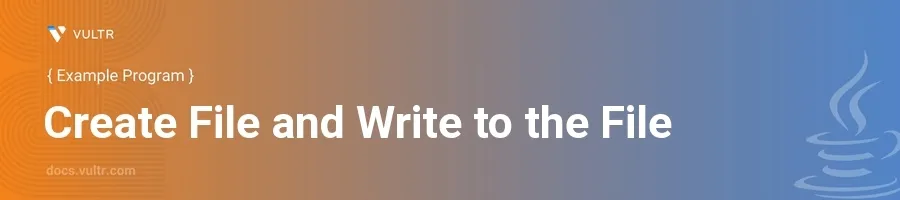
Introduction
The creation and management of files are foundational tasks in many software applications. Java, with its robust APIs, provides a straightforward method for these operations, ensuring developers can easily implement file handling in their projects. Understanding how to create and write to files is essential for storing data, logging, or simply saving configurations.
In this article, you will learn how to use Java to create a new file and write content to it. We will explore the use of essential classes such as File
, FileWriter
, and BufferedWriter
, and provide examples to demonstrate how these can be utilized to manage file operations effectively.
Creating a File in Java
Basic File Creation
Import the
File
class from thejava.io
package.Create an instance of the
File
class with a specified path for the new file.Use the
createNewFile
method to attempt file creation, and handle the potentialIOException
.javaimport java.io.File; import java.io.IOException; public class FileCreationExample { public static void main(String[] args) { try { File newFile = new File("newfile.txt"); if (newFile.createNewFile()) { System.out.println("File created: " + newFile.getName()); } else { System.out.println("File already exists."); } } catch (IOException e) { System.out.println("An error occurred while creating the file."); e.printStackTrace(); } } }
In this example, a new file named
newfile.txt
is created in the program's working directory. The program checks whether the file already exists to avoid overwriting an existing file. If an error occurs during file creation, it's caught and printed to the console.
Handling File Paths
Understand the differences between absolute and relative paths.
Specify a file path that fits the system’s directory structure.
It's important to differentiate between absolute and relative paths when specifying the file path:
- An absolute path specifies a file location from the root directory.
- A relative path specifies a file location relative to the current working directory of the application.
Writing to a File in Java
Using FileWriter and BufferedWriter
Import necessary classes from the
java.io
package.Create
FileWriter
andBufferedWriter
objects, passing the file path to write contents.Utilize the
write
method ofBufferedWriter
to write text to the file, and ensure to close the writers to flush and release system resources.javaimport java.io.FileWriter; import java.io.BufferedWriter; import java.io.IOException; public class WriteToFileExample { public static void main(String[] args) { try (BufferedWriter writer = new BufferedWriter(new FileWriter("newfile.txt", true))) { writer.write("Hello World!"); System.out.println("Successfully wrote to the file."); } catch (IOException e) { System.out.println("An error occurred while writing to the file."); e.printStackTrace(); } } }
This code snippet demonstrates using
FileWriter
andBufferedWriter
to append the string "Hello World!" to a file namednewfile.txt
. Thetrue
parameter in theFileWriter
constructor indicates that you want to append data to the file if it already exists. The try-with-resources statement ensures that both theFileWriter
andBufferedWriter
get closed properly after the operation completes.
Conclusion
Creating and writing files in Java can be accomplished effectively with standard I/O classes provided by the Java framework. By leveraging the functionality provided by File
, FileWriter
, and BufferedWriter
, you manage file operations with ease. Careful handling of file paths and exceptions ensures that your applications can securely and efficiently deal with file output, offering a robust way to handle data persistence. Implementing the outlined methods ensures your Java application can process and store data without any hurdles.
No comments yet.