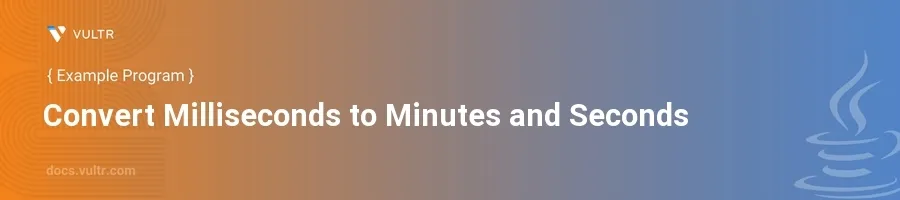
Introduction
Converting milliseconds into minutes and seconds is a common requirement in programming, especially when dealing with time measurements and performance tracking. This task can be approached by understanding how these time units relate: 1,000 milliseconds make up a second, and 60 seconds make up a minute.
In this article, you will learn how to effectively convert milliseconds into minutes and seconds using Java. Explore multiple examples which demonstrate the process using basic arithmetic operations and the modular arithmetic to achieve accurate conversions.
Basic Conversion Using Arithmetic Operations
Convert Milliseconds to Seconds and Minutes
Start with the number of milliseconds you want to convert.
Divide the milliseconds by 1,000 to convert it to seconds.
Use the result to find out how many full minutes are represented and what remains as seconds.
javalong milliseconds = 55000; // 55 seconds in milliseconds long totalSeconds = milliseconds / 1000; long minutes = totalSeconds / 60; long seconds = totalSeconds % 60; System.out.println(minutes + " minutes and " + seconds + " seconds");
In the above code,
55000
milliseconds convert into55
seconds. Further dividing and using modulo gives0
minutes and55
seconds.
More Detailed Conversion Example
Define a more complex time interval in milliseconds.
Apply the same conversion logic to determine minutes and seconds.
Print the results in a structured format.
javalong ms = 123456; // Example with more milliseconds long secondsFromMs = ms / 1000; long calculatedMinutes = secondsFromMs / 60; long remainingSeconds = secondsFromMs % 60; System.out.printf("Output: %d minutes and %d seconds%n", calculatedMinutes, remainingSeconds);
This code snippet converts
123456
milliseconds to2
minutes and3
seconds, following the conversion guidelines previously described.
Handling Larger Intervals
Converting Large Millisecond Values
Consider milliseconds that represent larger time spans, such as hours.
Compute the number of hours, minutes, and remaining seconds.
Print the findings effectively.
javalong largeMs = 5400000; // 1.5 hours in milliseconds long toSecs = largeMs / 1000; long hours = toSecs / 3600; long mins = (toSecs % 3600) / 60; long secs = (toSecs % 3600) % 60; System.out.printf("%d hours, %d minutes, and %d seconds%n", hours, mins, secs);
Here,
5400000
milliseconds is accurately converted into1
hour,30
minutes, and0
seconds. This example demonstrates the necessity to extend the conversion process to accommodate larger durations by including hours in the computation.
Edge Cases and Considerations
Zero and Negative Values: Test how the method behaves with edge values such as
0
or negative milliseconds.javalong edgeTest = 0; if (edgeTest < 0) { System.out.println("Invalid negative value"); } else { long sec = edgeTest / 1000; long minute = sec / 60; long remSeconds = sec % 60; System.out.printf("%d minutes and %d seconds%n", minute, remSeconds); }
This safeguard ensures that the program can handle unexpected or erroneous input by checking for negative values and reacting accordingly.
Performance: The arithmetic operations used are very fast and effective for conversions, but always validate with profiling if it's part of bigger, performance-sensitive applications.
Conclusion
Converting milliseconds to minutes and seconds in Java is straightforward using basic arithmetic operations. These methods ensure quick and accurate conversions, proving invaluable in applications that require precise time measurement. By following the examples and understanding their underlying principles, you can apply these conversions to various Java applications effectively, ensuring your programs handle time-based measurements reliably and efficiently.
No comments yet.