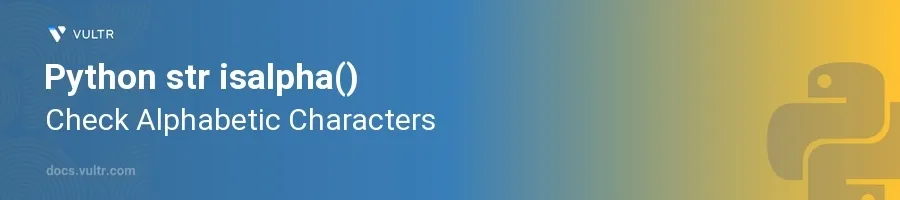
Introduction
The isalpha()
method in Python's string class is a straightforward yet powerful tool for checking whether a string contains only alphabetic characters. This method returns True
if all characters in the string are alphabetic (i.e., they are part of the alphabet used in English) and there is at least one character; otherwise, it returns False
. Its utility is evident in various applications, from data validation to processing textual data where alphabetic content needs to be ascertained.
In this article, you will learn how to use the isalpha()
method effectively. Explore how this method can serve as a critical tool for string handling, ensuring input validity in user data, and filtering textual information in data analysis.
Checking Strings with isalpha()
Basic Usage of isalpha()
Start with a basic string.
Apply the
isalpha()
method.pythonexample = "Hello" result = example.isalpha() print(result)
This code returns
True
because all characters in the string"Hello"
are alphabetic.
Handling Strings with Spaces
Create a string containing alphabetic characters and spaces.
Use the
isalpha()
method to check the string.pythongreeting = "Hello World" result = greeting.isalpha() print(result)
Here,
isalpha()
returnsFalse
because the string contains a space alongside alphabetic characters.
Mixed Content Strings
Consider a string that includes numbers and alphabetic characters.
Check the string with
isalpha()
.pythonalphanumeric = "abc123" result = alphanumeric.isalpha() print(result)
In this scenario, because the string includes numerals,
isalpha()
evaluates toFalse
.
Use Cases in Data Validation and Processing
Ensuring Alphabetic User Inputs
Validate that a user's name input is strictly alphabetic.
pythonuser_input = "JohnDoe" if user_input.isalpha(): print("Valid name") else: print("Name should only contain alphabetic characters.")
This snippet helps ensure that a name entered in a form or application contains only alphabetic characters.
Filtering Textual Data in Lists
Filter a list to include items that are only alphabetic.
pythonnames = ["Alice", "1234", "Bob3", "Carol"] alphabetic_names = [name for name in names if name.isalpha()] print(alphabetic_names)
This list comprehension returns a new list
['Alice', 'Carol']
, containing only the valid alphabetic items from the original list.
Conclusion
The isalpha()
method in Python is essential for verifying if a string consists exclusively of alphabetic characters. Its application ranges from validating user input in forms to filtering and processing textual data in larger datasets. By employing the techniques discussed, improve data integrity and efficiency in your Python scripts, ensuring they handle text-based data accurately and effectively.
No comments yet.