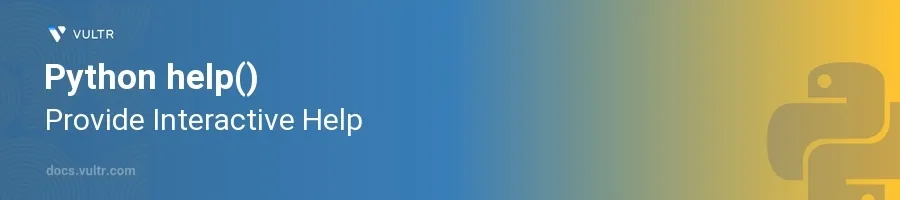
Introduction
The help()
function in Python is an invaluable tool for both beginners and experienced developers, offering instant, interactive access to Python's extensive documentation directly from the interpreter. Whether you're unsure about how to use a particular module, class, method, or function, help()
can provide the insights you need without leaving your development environment.
In this article, you will learn how to leverage the help()
function to explore Python documentation conveniently. Discover how to use this tool to better understand Python's built-in features, external libraries, and even your custom modules.
Understanding the help() Function
Basics of Using help()
Invoke the
help()
function without any arguments to enter the interactive help utility.pythonhelp()
This command brings up the interactive help system, which allows you to browse topics, modules, keywords, and more.
Type
quit
or press Control-D to exit the interactive help.
Querying Specific Modules or Functions
Pass the name of a module, function, or variable to
help()
to get detailed documentation directly.pythonimport os help(os)
This code displays the full documentation for the
os
module, detailing functions, classes, and attributes contained within the module.Search for help on a specific function or method within a module:
pythonimport json help(json.load)
This command provides detailed documentation for the
json.load
function, showing its purpose, parameters, and usage examples.
Using help() with Custom Objects
Define a class or function with docstrings.
Use
help()
on your custom classes or functions to see the documentation provided in the docstring.pythonclass MyCalculator: """ A simple calculator class to demonstrate help functionality. Methods: add(a, b) - Returns the sum of a and b. subtract(a, b) - Returns the difference between a and b. """ def add(a, b): """ Adds two numbers a and b. """ return a + b def subtract(a, b): """ Subtracts b from a. """ return a - b help(MyCalculator)
Reading this code, you'll see the documentation for
MyCalculator
as defined in its docstring. This includes descriptions of the class and its methods.
Exploring Libraries Beyond Standard Library
Install third-party libraries using pip, for example,
pip install pandas
.Use
help()
to explore these libraries just as you would with built-in modules.pythonimport pandas as pd help(pd.DataFrame)
The
help()
function will provide comprehensive details about theDataFrame
class in thepandas
library, helpful for understanding its usage and methods.
Conclusion
The help()
function in Python stands as a robust and easy-to-use tool for accessing Python's comprehensive documentation system. It greatly simplifies the learning process for new functions, methods, and libraries. Whether working with Python's standard library or exploring third-party packages, help()
proves indispensable in quickly grasping how different parts of Python and its vast ecosystem are supposed to be used. Implement this in your day-to-day coding practices to diminish downtime and enhance your productivity and code quality.
No comments yet.