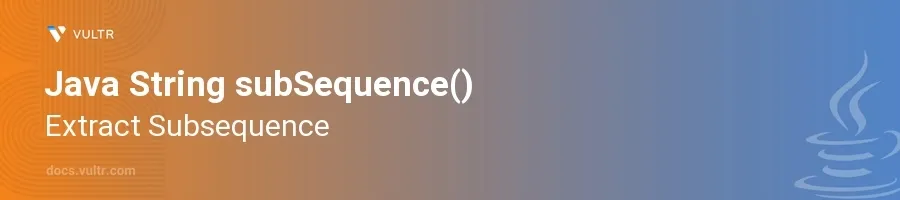
Introduction
The subSequence()
method in Java's String
class is a versatile tool for extracting a substring from a given string. This method is particularly useful when manipulating text data, allowing developers to easily access parts of strings based on specified indexes. It returns a character sequence rather than a new string object, which is crucial for memory management, especially in large-scale applications where string manipulation is frequent.
In this article, you will learn how to leverage the subSequence()
method in various ways to extract subsequences from strings. Explore how this technique can be applied to data validation, slicing string data for processing, and even in complex string manipulation tasks.
Understanding the subSequence() Method
Basic Usage of subSequence()
Begin with a string from which you want to extract a subsequence.
Use the
subSequence()
method by providing the start and end indices.javaString str = "Hello, world!"; CharSequence result = str.subSequence(7, 12); System.out.println(result);
Here, the method extracts characters starting from index 7 up to, but not including, index 12. The output will be
"world"
.
Handling Index Out of Bound Errors
Recognize the risk of index out of bounds exceptions when indices are incorrectly specified.
Always check the length of the string before attempting to extract a subsequence.
javaString str = "Short"; if (str.length() >= 5) { CharSequence result = str.subSequence(0, 5); System.out.println(result); } else { System.out.println("String too short for the requested subsequence."); }
This code first checks if the string length is sufficient to handle the requested indices. It prints "Short" if valid, and provides an error message if not.
Advanced Applications of subSequence()
Dynamic Substring Extraction
Utilize
subSequence()
in dynamic conditions, such as parsing user input where the substring length can vary.Implement a method that adjusts based on the input length or specific markers.
javaString userInput = "Name:John Doe"; int start = userInput.indexOf(":") + 1; CharSequence name = userInput.subSequence(start, userInput.length()); System.out.println(name);
This example dynamically finds the start of the name following the colon and extracts it, regardless of the name length. The output will be
"John Doe"
.
Combining subSequence() with Other String Methods
Combine
subSequence()
with other string methods to perform complex manipulations.Example: Remove whitespace and extract a portion of a cleaned-up string.
javaString original = " Data: 12345 "; String trimmed = original.trim(); CharSequence data = trimmed.subSequence(6, trimmed.length()); System.out.println(data);
This snippet first removes the leading and trailing whitespaces using
trim()
and then extracts the numerical data. The output will be"12345"
.
Conclusion
The subSequence()
method in Java's String
class is a powerful function that facilitates robust string processing tasks. By understanding how to effectively use this method, you can handle and manipulate strings more precisely in your applications. Whether extracting simple substrings or implementing more complex string operations, subSequence()
offers a reliable and efficient way to process textual data. Harnessing these capabilities ensures your Java applications are well-equipped to manage diverse string manipulation needs efficiently.
No comments yet.