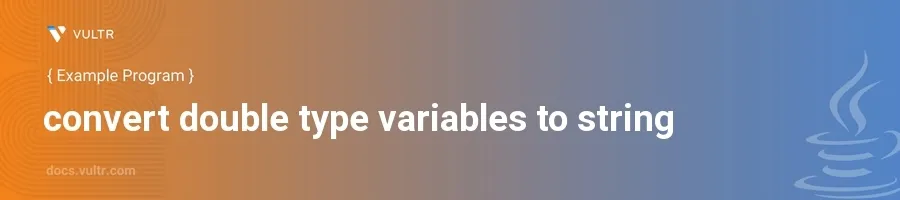
Introduction
Converting data types in Java is a common operation, especially when it involves primordial data types like double
and String
. This capability is crucial in applications where numeric values need to be displayed as part of GUIs or need to be concatenated with other string data for output or logging purposes.
In this article, you will learn how to convert a double
type variable to a String
in Java. The examples provided will guide you through various methods to achieve this conversion, ensuring you can select the most appropriate approach depending on your specific application needs.
Basic Conversion Using toString()
Convert Using the Double Class
Use the
Double.toString()
static method to convert a double value directly to a String.javadouble doubleValue = 9.5; String stringValue = Double.toString(doubleValue); System.out.println(stringValue);
This method utilizes the
Double
class's static methodtoString()
, which takes a double value as an argument and returns its string representation. The output of the code snippet above would be the string "9.5".
Using String.valueOf()
Employ the
String.valueOf()
method for conversion, which is often used for its versatility with different data types.javadouble anotherDoubleValue = 123.45; String anotherStringValue = String.valueOf(anotherDoubleValue); System.out.println(anotherStringValue);
String.valueOf()
is a static method that can handle various data types, including double, int, long, and more. It returns a string representation of the passed argument, as shown above where "123.45" is the output.
Formatting Double Values Before Conversion
Using DecimalFormat
Use
DecimalFormat
to format the double value before converting it to a string. This is useful when needing specific decimal point precision or patterns.javadouble largeDoubleValue = 1234567.8910; DecimalFormat formatter = new DecimalFormat("#,##0.00"); String formattedString = formatter.format(largeDoubleValue); System.out.println(formattedString);
DecimalFormat
allows for the creation of a custom numeric format. In this example, the format "#,##0.00" includes comma separators for thousands and exactly two decimal places, converting the double to "1,234,567.89".
Using String Formatting
Utilize
String.format()
for converting and formatting in a single step.javadouble piValue = 3.14159265359; String formattedPiString = String.format("%.2f", piValue); System.out.println(formattedPiString);
This statement makes use of Java’s
String.format()
method, where "%.2f" specifies that the double should be formatted to two decimal places. The output here is "3.14", demonstrating a straightforward way to tailor the output precision.
Converting Double Arrays to String Arrays
Iterative Conversion
Convert each element of a double array to a string array manually using a loop.
javadouble[] doubleArray = {0.1, 1.1, 2.2, 3.3}; String[] stringArray = new String[doubleArray.length]; for(int i = 0; i < doubleArray.length; i++) { stringArray[i] = String.valueOf(doubleArray[i]); } // Output the String array System.out.println(Arrays.toString(stringArray));
This example demonstrates how to iterate over a double array and convert each element to a string using
String.valueOf()
. The array's string representation "[0.1, 1.1, 2.2, 3.3]" gets printed, showcasing individual double values converted to strings.
Conclusion
Transformation of double values to strings in Java can be done using several methods, each tailored to fit different scenarios—whether converting straightforward numeric values or formatting numbers for readability. Your choice of conversion method depends on the requirements for format and computation overhead. The skills covered in this article help integrate numbers within text-based applications effectively and elegantly, enhancing both functionality and user interaction.
No comments yet.