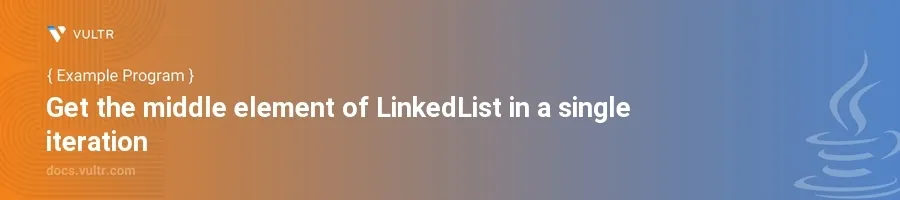
Introduction
When working with linked lists in Java, accessing the middle element quickly and efficiently can be crucial, especially in performance-sensitive applications. Unlike arrays, linked lists do not offer direct access to their elements, making them challenging to manage optimally for this kind of operation. This article explores a specific method to retrieve the middle element of a singly linked list in just one pass through the list.
In this article, you will learn how to craft a Java program to determine the middle element of a singly linked list using a single iteration. This efficient approach utilizes the fast and slow pointer technique, often known as the "tortoise and hare" algorithm.
Understanding the Problem
The challenge is to find the middle element of a linked list in one iteration. Given that a linked list's elements are not indexed, traditional direct access methods are not applicable. The solution needs to traverse the list in a way that allows identification of the middle element by the time the traversal is completed.
Core Principle: Tortoise and Hare Algorithm
The algorithm uses two pointers:
- Slow pointer - Moves one node at a time.
- Fast pointer - Moves two nodes at a time.
By the time the fast pointer reaches the end of the list, the slow pointer will be at the middle. This technique efficiently pinpoints the middle in a single pass.
Implementing the Solution in Java
To put this theory into practice, follow the outlined steps to construct a robust Java program. You will define a LinkedList
class and utilize the tortoise and hare technique.
Define a Simple Linked List Node Class
Begin by defining a basic node class that represents the elements of the linked list.
javaclass Node { int data; Node next; public Node(int data) { this.data = data; this.next = null; } }
Develop the LinkedList Class
In this step, create a linked list class with essential functionalities such as adding elements and finding the middle element.
javaclass LinkedList { Node head; public void add(int data) { Node newNode = new Node(data); newNode.next = head; head = newNode; } public int getMiddleElement() { Node slow = head; Node fast = head; while (fast != null && fast.next != null) { slow = slow.next; fast = fast.next.next; } return slow.data; } }
In
getMiddleElement()
, the slow pointer advances one node for every two nodes the fast pointer advances. As soon as the fast pointer cannot advance (i.e.,fast.next
is null because it's at the end of the list or no nodes remain), the slow pointer points to the middle node.
Example Main Class to Demonstrate Usage
Finally, put together a main class to test the functionality of your LinkedList:
javapublic class Main { public static void main(String[] args) { LinkedList myList = new LinkedList(); myList.add(5); myList.add(4); myList.add(3); myList.add(2); myList.add(1); // Constructed list is 1 -> 2 -> 3 -> 4 -> 5 System.out.println("The middle element is: " + myList.getMiddleElement()); } }
This example initializes the list and uses
add()
to populate it. Upon callinggetMiddleElement()
, it prints the middle of the list.
Conclusion
Using the tortoise and hare algorithm, you can efficiently find the middle element of a linked list in a single iteration. This Java program demonstrates a practical approach to this common data structure challenge. The method ensures you work within the constraints of the linked list’s linear nature while maintaining optimal performance. Implement this strategy in scenarios where quick access to central elements is necessary, enhancing both speed and efficiency in your data handling tasks.
No comments yet.