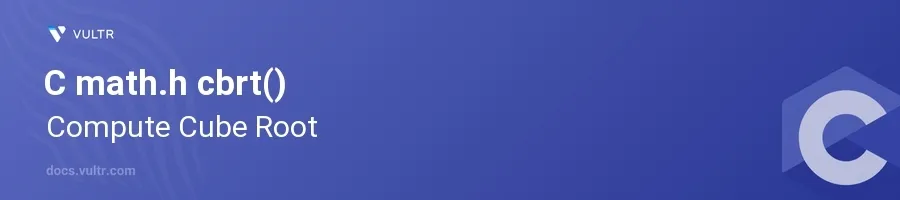
Introduction
The cbrt()
function from C's math.h
library computes the cube root of a given number. This mathematical function is integral in various engineering, physics, and graphics computations where determining the volume or scaling objects uniformly is necessary.
In this article, you will learn how to apply the cbrt()
function to compute the cube roots of various numbers. You'll explore its usage through straightforward examples and understand how to integrate it into your C programs.
Understanding the cbrt() Function
The cbrt()
function requires a single argument and returns the cube root of that argument as a double. It's essential to include the math.h
header file in your C source to utilize this function.
Basic Usage of cbrt()
Import the
math.h
library to your C program.Define a variable to hold the number for which you want the cube root.
Apply
cbrt()
and store or display the result.c#include <stdio.h> #include <math.h> int main() { double num = 27.0; double cube_root = cbrt(num); printf("The cube root of %.2f is %.2f\n", num, cube_root); return 0; }
This code snippet calculates the cube root of 27.0. When executed, it will print "The cube root of 27.00 is 3.00".
Handling Negative Numbers
Understand that
cbrt()
correctly handles negative numbers as well.Pass a negative number to
cbrt()
and observe the result.c#include <stdio.h> #include <math.h> int main() { double num = -8.0; double cube_root = cbrt(num); printf("The cube root of %.2f is %.2f\n", num, cube_root); return 0; }
In this example, the code computes the cube root of -8.0, resulting in -2.0. This demonstrates
cbrt()
's ability to handle and return accurate results for negative inputs.
Using cbrt() with Variables
Use variables to perform calculations or conditions before applying
cbrt()
.Define a variable, perform operations, and then compute the cube root.
c#include <stdio.h> #include <math.h> int main() { double volume = 216.0; // example of a calculated volume double side_length = cbrt(volume); printf("Side length to form a cube of volume %.2f is %.2f\n", volume, side_length); return 0; }
This program calculates the side length of a cube given its volume. It uses
cbrt()
to determine the length of a side that would make up a cube of 216.0 cubic units.
Conclusion
The cbrt()
function in C provides an efficient way to compute cube roots, an operation frequently required in scientific, engineering, and graphical programming. It handles both positive and negative numbers effectively and integrates seamlessly into C programs. Utilize this function to easily compute cube roots in your projects, helping to simplify complex calculations and enhance the functionality of your programs. Through the examples provided, enhance your ability to work with mathematical functions in C, ensuring robust and accurate computations.
No comments yet.