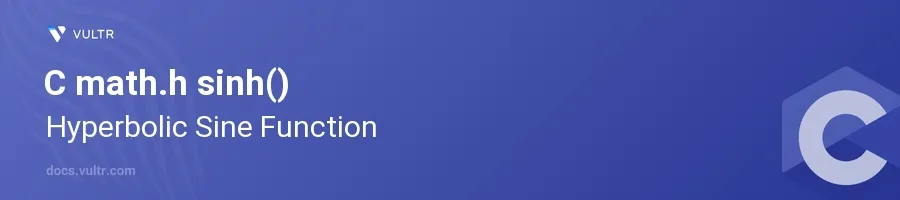
Introduction
The hyperbolic sine function, represented by sinh()
, is a function from the C standard library math.h
that calculates the hyperbolic sine of a given angle, expressed in radians. This mathematical function is essential in various scientific and engineering computations, especially where hyperbolic functions apply, such as in the modeling of waves or in certain calculus problems.
In this article, you will learn how to utilize the sinh()
function in C programming. Explore working examples that will help you understand how to apply this function in practical programming tasks involving mathematical calculations.
Understanding the sinh() Function
Basic Usage of sinh()
Include the
math.h
library to use thesinh()
function.Pass a double type argument to
sinh()
, representing the angle in radians, and store the result.c#include <stdio.h> #include <math.h> int main() { double angle = 1.0; // angle in radians double result = sinh(angle); printf("The hyperbolic sine of %lf is %lf\n", angle, result); return 0; }
This code calculates the hyperbolic sine of 1 radian and prints the result. The
sinh()
function returns the hyperbolic sine of the given angle.
Exploring sinh() with Different Inputs
Test
sinh()
with varying angles to see different outputs.Prepare a loop to auto-generate angles and compute their hyperbolic sines.
c#include <stdio.h> #include <math.h> int main() { for (double angle = 0.0; angle <= 2.0; angle += 0.1) { double result = sinh(angle); printf("The hyperbolic sine of %lf is %lf\n", angle, result); } return 0; }
This loop increments the angle from 0 to 2 radians in steps of 0.1 and computes the hyperbolic sine for each value, displaying the result. This helps in understanding how the
sinh()
function behaves over a range of values.
Handling Special Cases
Consider edge cases like zero and very large numbers to evaluate
sinh()
behavior.Code these examples to examine and verify their outputs.
c#include <stdio.h> #include <math.h> int main() { double result_zero = sinh(0); // should return 0 double result_large = sinh(1000); // explore large value behavior printf("The hyperbolic sine of 0 is %lf\n", result_zero); printf("The hyperbolic sine of a large number (1000 radians) is %lf\n", result_large); return 0; }
For
sinh(0)
, the expected result is0
. For extremely large values such as1000
radians, the function will demonstrate the rapid growth characteristic of hyperbolic functions.
Conclusion
Utilizing the sinh()
function from the C math.h
library allows you to calculate the hyperbolic sine of an angle in radians efficiently. Whether you are handling simple cases or exploring complex calculations involving a range of values, sinh()
provides accurate results useful in numerous scientific, engineering, or mathematical applications. Familiarize yourself with its behavior in different scenarios to fully harness its capabilities in your projects.
No comments yet.