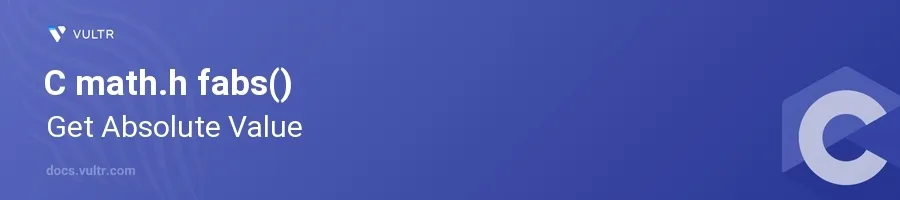
Introduction
The fabs()
function from the C standard library's math.h
header file is used to compute the absolute value of a floating-point number. This function is essential in various programming scenarios, particularly in fields requiring precise mathematical calculations, such as engineering, physics, and computer graphics.
In this article, you will learn how to effectively use the fabs()
function to obtain the absolute value of floating-point numbers. Explore practical examples illustrating its usage in different contexts and discover how it handles various types of input values.
Utilizing fabs() Function
Obtain Absolute Value of a Single Float
Include the
math.h
header file to accessfabs()
.Define a floating-point variable with a negative value.
Use
fabs()
to compute the absolute value.Print the result.
c#include <stdio.h> #include <math.h> int main() { double value = -23.45; double absValue = fabs(value); printf("The absolute value of %.2f is %.2f\n", value, absValue); return 0; }
This code snippet demonstrates obtaining the absolute value of the
value
variable. The functionfabs()
correctly converts-23.45
to23.45
.
Handling Positive and Zero Values
Initialize variables with positive and zero values.
Apply
fabs()
to these values.Output the results to verify correctness.
c#include <stdio.h> #include <math.h> int main() { double positiveValue = 54.76; double zeroValue = 0.0; printf("Absolute value of %.2f is %.2f\n", positiveValue, fabs(positiveValue)); printf("Absolute value of %.2f is %.2f\n", zeroValue, fabs(zeroValue)); return 0; }
In this example,
fabs()
processes both positive and zero values. The output shows the function retains the positive number and confirms zero remains unchanged.
Comparing fabs() with Floating Point and Integer Types
Understand that
fabs()
is specifically for floating-point numbers.Use both
float
anddouble
values to demonstratefabs()
usage.Observe how implicit casting from
int
tofloat
ordouble
might be required.c#include <stdio.h> #include <math.h> int main() { float floatValue = -123.456f; double doubleValue = -789.0123; int intValue = -100; printf("Absolute of float: %.3f\n", fabs(floatValue)); printf("Absolute of double: %.4f\n", fabs(doubleValue)); printf("Absolute of int (casted to double): %.1f\n", fabs((double)intValue)); return 0; }
This code sample illustrates the functionality of
fabs()
with various data types, highlighting the need for casting integer types explicitly.
Conclusion
The fabs()
function in C's math.h
library is a robust tool for determining the absolute value of floating-point numbers. By integrating fabs()
into your programming toolkit, you enhance your capability to perform precise mathematical calculations unaffected by the sign of the numbers. The examples provided here serve as a foundation for implementing this function in diverse programming scenarios, allowing more efficient and readable numeric computations in your projects.
No comments yet.