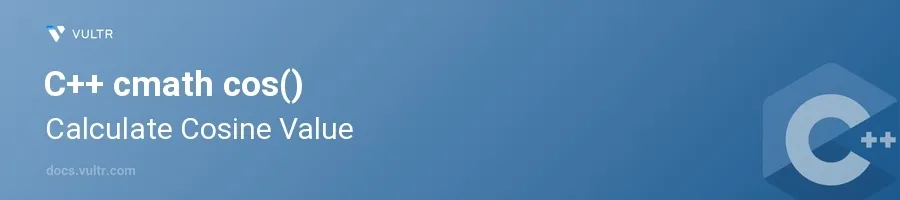
Introduction
The cos()
function in C++ is part of the <cmath>
header and is utilized to calculate the cosine of a given angle, which is expressed in radians. This trigonometric function is fundamental in various fields such as physics, engineering, and computer graphics for performing rotational transformations and calculations.
In this article, you will learn how to effectively use the cos()
function to calculate cosine values in C++. This guide covers basic usage, demonstrates handling of different data types, and explores practical examples to integrate cosine calculations into your applications.
Using cos() Function in C++
Basic Usage of cos()
Include the
<cmath>
header in your C++ program.Declare a variable to store the angle in radians.
Apply the
cos()
function and store the result.cpp#include <cmath> #include <iostream> int main() { double angle = M_PI / 4; // 45 degrees expressed in radians double cosine_value = cos(angle); std::cout << "Cosine of 45 degrees: " << cosine_value << std::endl; }
This code calculates the cosine of 45 degrees. Note that the angle must be in radians, not degrees.
M_PI
is a constant in<cmath>
representing the value of pi, which helps convert degrees to radians.
Handling Different Data Types
Understand that
cos()
can accept any floating-point type:float
,double
, orlong double
.Use
cos()
function with different data types and observe the precision.cpp#include <cmath> #include <iostream> int main() { // Using float float angle_f = M_PI / 4; float result_f = cos(angle_f); std::cout << "Float cos: " << result_f << std::endl; // Using double double angle_d = M_PI / 4; double result_d = cos(angle_d); std::cout << "Double cos: " << result_d << std::endl; // Using long double long double angle_ld = M_PI / 4; long double result_ld = cos(angle_ld); std::cout << "Long double cos: " << result_ld << std::endl; }
In this example,
cos()
is used with three different floating-point types. The output demonstrates how the precision of the result can vary depending on the data type.
Practical Application Example
Apply the
cos()
function in a practical scenario, such as calculating the horizontal component of a vector at a specific angle.Implement the calculation.
cpp#include <cmath> #include <iostream> int main() { double angle = M_PI / 3; // 60 degrees in radians double magnitude = 10; // The magnitude of the vector double horizontal_component = magnitude * cos(angle); std::cout << "Horizontal component: " << horizontal_component << std::endl; }
In this code, calculate the horizontal component of a vector given its magnitude and an angle. This method is vital in physics for resolving forces or velocities into their components.
Conclusion
The cos()
function from the <cmath>
library in C++ is invaluable for calculating the cosine of angles in radians. It enables precision across different floating-point types and is critical in scientific computations where trigonometric functions are frequent. By incorporating cos()
in your C++ toolkit, you facilitate complex geometrical transformations and analyses with ease and accuracy. Through the examples provided, you can confidently integrate cosine calculations into your projects, enhancing both functionality and numerical precision.
No comments yet.