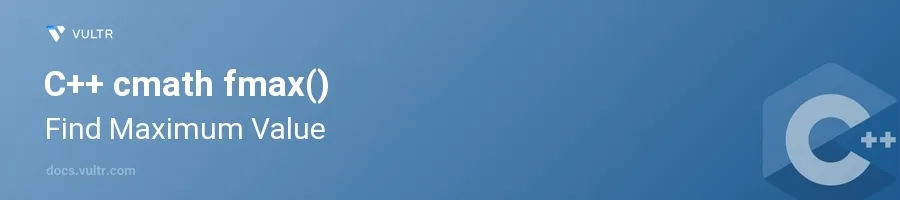
Introduction
The fmax()
function in C++ is a part of the <cmath>
header and serves a key role in determining the greater of two floating-point numbers. This function is particularly crucial when you need precise and efficient calculations in critical applications such as financial analytics, scientific computing, or any scenario requiring the comparison of two numerical values.
In this article, you will learn how to effectively harness the fmax()
function in various programming contexts. Explore how this function can optimize your code by ensuring that you always retrieve the maximum value between two numbers, even in edge cases involving special floating-point values like NaN (Not a Number).
Understanding fmax()
The fmax()
function is straightforward to use but understanding its behavior with different types of inputs is essential for proper implementation.
Basic Usage of fmax()
Include the
<cmath>
header in your C++ code, asfmax()
is part of this library.Call
fmax()
with two floating-point arguments.cpp#include <iostream> #include <cmath> int main() { double num1 = 3.5; double num2 = 4.5; double max_value = fmax(num1, num2); std::cout << "Maximum value is: " << max_value << std::endl; }
This code snippet compares two double values (
num1
andnum2
) and prints the greater number,4.5
, using thefmax()
function.
Handling Special Values
Become aware that
fmax()
is especially useful when handling NaN values.Recognize its capability to return a non-NaN value if one of the operands is a NaN.
cpp#include <iostream> #include <cmath> int main() { double num1 = nan(""); double num2 = 5.5; double max_value = fmax(num1, num2); std::cout << "Maximum value when one is NaN: " << max_value << std::endl; }
In this example, the output will be
5.5
asfmax()
successfully bypasses the NaN value and likely returns the non-NaN number.
Usage with Negative Numbers
Note that
fmax()
also works correctly with negative numbers.Use it to determine the maximum between negative values.
cpp#include <iostream> #include <cmath> int main() { double neg1 = -10.0; double neg2 = -20.0; double max_value = fmax(neg1, neg2); std::cout << "Maximum of negative numbers: " << max_value << std::endl; }
Even with negative numbers,
fmax()
correctly determines-10.0
as the maximum value in the above snippet.
Conclusion
The fmax()
function in C++ offers a robust and reliable way to find the maximum value between two floating-point numbers, ensuring correct results even in complex scenarios involving negative values or NaNs. With the practical examples shown, adopt fmax()
in your numerical comparisons to simplify your coding tasks and enhance the reliability of your computations. Leverage this function to maintain clarity and efficiency in your mathematical operations, making your C++ programs more precise and easier to maintain.
No comments yet.