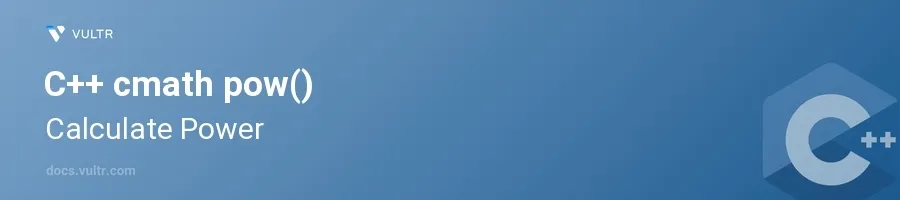
Introduction
The pow()
function in C++ is part of the cmath
library and is specifically designed to compute the power of a number. This function provides an efficient way to raise a base to the power of an exponent, which is a common operation in various mathematical and scientific computations.
In this article, you will learn how to effectively utilize the pow()
function to calculate powers of numbers. The focus will be on understanding how this function works with various data types and scenarios, ensuring you are equipped to handle basic to complex power calculations in C++.
Understanding the pow() Function
Basic Usage of pow()
Include the
cmath
library in your C++ program.Use the function
pow(base, exponent)
wherebase
andexponent
are the numbers you are working with.cpp#include <iostream> #include <cmath> int main() { double base = 2.0; double exponent = 3.0; double result = pow(base, exponent); std::cout << "2 to the power of 3 is " << result << std::endl; }
This snippet calculates (2^3), which results in 8.
pow()
returns a double value representing the result of raisingbase
to the power ofexponent
.
Handling Negative Exponents
Understand that
pow()
can also handle negative exponents, which means taking the reciprocal of the base raised to the absolute value of the exponent.Apply
pow()
with a negative exponent.cpp#include <iostream> #include <cmath> int main() { double base = 2.0; double exponent = -2.0; double result = pow(base, exponent); std::cout << "2 raised to the power of -2 is " << result << std::endl; }
In this case,
pow()
computes (2^{-2}), or (\frac{1}{4}), and returns 0.25.
Using pow() with Integer Types
Note that even though integers are used as arguments,
pow()
converts them to double internally.Perform a power operation where the base or exponent is an integer.
cpp#include <iostream> #include <cmath> int main() { int base = 3; int exponent = 4; double result = pow(base, exponent); std::cout << "3 raised to the power of 4 is " << result << std::endl; }
The output here is (3^4), calculated as 81.0. Despite both
base
andexponent
being integers, the result is returned as a double for precision.
Edge Cases to Consider
Contemplate the result when the
base
is zero andexponent
is positive.Consider special cases like zero raised to the power of zero.
cpp#include <iostream> #include <cmath> int main() { double result1 = pow(0, 5); double result2 = pow(0, 0); std::cout << "0 to the power of 5 is " << result1 << std::endl; std::cout << "0 to the power of 0 is " << result2 << std::endl; // Typically defined as 1 in math libraries }
The first calculation returns 0, as any non-zero number raised to the power of zero results in 1. The second scenario, where (0^0) is often defined as 1 by many mathematical software and libraries.
Conclusion
Utilize the pow()
function in C++ to effectively and accurately perform power calculations. From handling simple to complex scenarios, this function supports both integer and floating-point arguments, providing flexibility and precision in mathematical computations. Implement the techniques discussed to enhance computational tasks in your C++ projects, ensuring both clarity and efficiency in your code.
No comments yet.